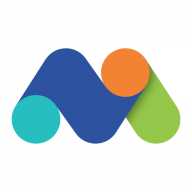
Matomo
AnalyticsUsing the Matomo Analytics API
If you have a website and want to track your visitor statistics, Matomo provides an Analytics API that allows you to get almost any data about your website visitors and their behavior. You can use Matomo Analytics API to integrate Matomo data into any application or tool.
The Matomo Analytics API uses REST calls to send and receive data. In this blog post, we'll walk through a few examples of how to use the Matomo Analytics API, using JavaScript code.
Examples of Matomo Analytics API calls
Real-time number of visitors on a website
The following JavaScript code gets the number of visitors that are currently on your website in real-time.
var siteId = 1; // Your Matomo Site ID (replace 1 with your actual site ID)
var apiUrl = 'https://matomo.example.com/index.php';
var authToken = 'YOUR_AUTH_TOKEN'; // Your Matomo auth token (check your Matomo user profile for your auth token)
var url = apiUrl + '?module=API&method=Live.getCounters&idSite=' + siteId + '&format=JSON&token_auth=' + authToken;
fetch(url)
.then(response => response.json())
.then(data => {
console.log('Number of visitors: ' + data[0]['visitors']);
});
Get the top pages of your website
The following JavaScript code gets the top pages of your website, ranked by the number of visits.
var siteId = 1; // Your Matomo Site ID (replace 1 with your actual site ID)
var apiUrl = 'https://matomo.example.com/index.php';
var authToken = 'YOUR_AUTH_TOKEN'; // Your Matomo auth token (check your Matomo user profile for your auth token)
var url = apiUrl + '?module=API&method=Actions.getPageUrls&format=JSON&idSite=' + siteId + '&period=day&date=today&expanded=1&token_auth=' + authToken;
fetch(url)
.then(response => response.json())
.then(data => {
console.log('Top Pages:');
data.forEach(page => {
console.log(page.label + ': ' + page.nb_visits);
});
});
Get the location of your website visitors
The following JavaScript code gets the location of your website visitors.
var siteId = 1; // Your Matomo Site ID (replace 1 with your actual site ID)
var apiUrl = 'https://matomo.example.com/index.php';
var authToken = 'YOUR_AUTH_TOKEN'; // Your Matomo auth token (check your Matomo user profile for your auth token)
var url = apiUrl + '?module=API&method=UserCountry.getCountry&format=JSON&idSite=' + siteId + '&period=day&date=today&token_auth=' + authToken;
fetch(url)
.then(response => response.json())
.then(data => {
console.log('Visitors by Country:');
data.forEach(country => {
console.log(country.label + ': ' + country.nb_visits);
});
});
Conclusion
The Matomo Analytics API provides a robust API for integrating your Matomo data into any app or tool. With the examples above, you can start exploring the data available to you and building custom applications.