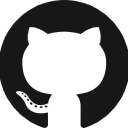
iDigBio
ScienceAccessing the iDigBio Search API with JavaScript
iDigBio is an online repository for biological specimen data, providing public access to more than 155 million records. The iDigBio Search API is a publicly available interface for querying this data programmatically. In this post, we will guide you through the process of accessing the iDigBio Search API using JavaScript and provide you with several API example codes.
Requirements
- You will need a basic understanding of JavaScript to follow this tutorial.
- You should also have an iDigBio account, as you will need an API key to access the API.
Getting Started
- First, navigate to https://github.com/idigbio/idigbio-search-api/wiki.
- Review the "Quick Start" guide to understand the overall process of accessing iDigBio data via the Search API.
- Scroll down to the "Query Structure" section in the left-hand menu to see the details of what kind of queries you can perform.
- Optionally, familiarize yourself with the "Search Filters" section to learn about additional parameters you can use to refine your search queries.
Using JavaScript to Access the API
Once you have an iDigBio login and API key, you can use the following JavaScript code to access the API:
async function search_iDigBio(query) {
const response = await fetch(`https://search.idigbio.org/v2/search/records?q=${query}&apiKey=${API_KEY}`);
const data = await response.json();
return data;
}
In this code, query
is the search query parameter and API_KEY
is your API key. The function sends a GET request to the iDigBio Search API and returns the JSON response.
Example Query
Below is an example query that searches for records of the bird species "Aix sponsa" occurring in Florida, USA.
const query = [
`scientificname:"Aix sponsa"`,
`country:USA`,
`stateprovince:Florida`
].join(' AND ');
search_iDigBio(query).then(data => {
console.log(data);
});
This query uses the scientificname
, country
, and stateprovince
search filters to refine the search results.
Conclusion
By following the steps outlined in this post, you now know how to access the iDigBio Search API and perform custom queries using JavaScript. With this knowledge, you can explore the vast amounts of publicly available biological specimen data provided by iDigBio.