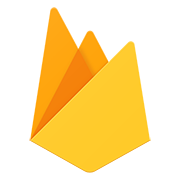
Landmark Recognition
Machine LearningHow to recognize landmarks with the Firebase ML Kit API
If you're building a web or mobile app that involves recognizing landmarks, you're in luck! Firebase provides the ML Kit API for recognizing landmarks with machine learning. Here's how to use it in JavaScript.
First, you'll need to set up a Firebase project and enable the ML Kit API. Follow the instructions here: https://firebase.google.com/docs/ml-kit/get-started.
Next, you'll need to import the Firebase ML Kit API into your JavaScript project. Here's an example:
import { initializeApp } from 'firebase/app';
import { getLandmark } from 'firebase/mlkit';
const config = {
// your Firebase project config goes here
};
const app = initializeApp(config);
const landmarkRecognizer = getLandmark(app);
Now you have access to the getLandmark
method, which takes an image file and returns a promise containing information about the recognized landmark. Here's an example:
const fileInput = document.getElementById('file-input');
fileInput.addEventListener('change', async () => {
const file = fileInput.files[0];
try {
const result = await landmarkRecognizer.detectLandmark(file);
console.log(result);
} catch (error) {
console.error(error);
}
});
In this example, we're using an input
element with the type="file"
attribute to let the user select an image file. When the user selects a file, we pass it to the detectLandmark
method of the landmarkRecognizer
object. This method returns a promise that resolves to an object with information about the recognized landmark, including its name, geographical coordinates, and confidence level.
That's it! With just a few lines of JavaScript code, you can use the Firebase ML Kit API to recognize landmarks in your web or mobile app. Happy coding!