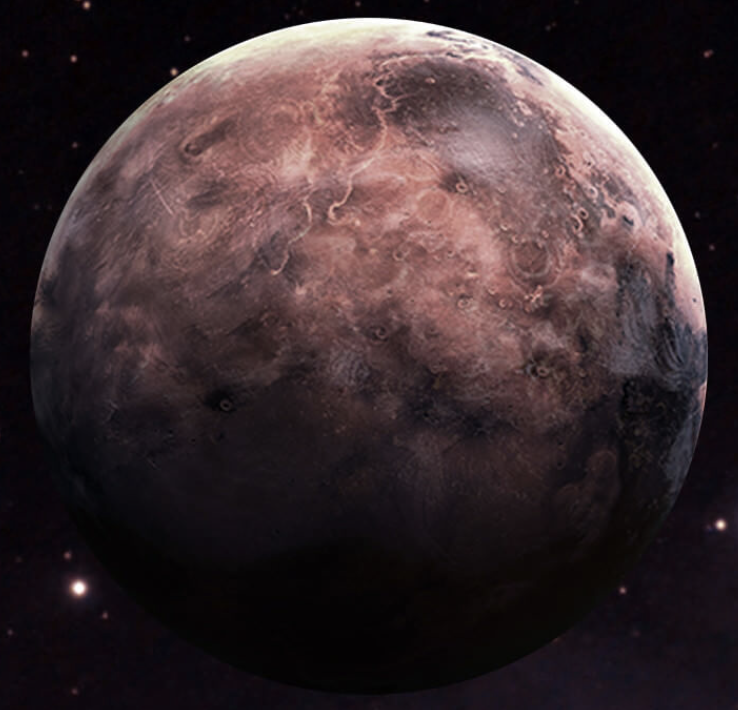
Mercury Retrograde API
ScienceYou may have heard friends say, “Oh, no – Mercury is about to retrograde again!” If you don’t know astrology, you may have wondered what they were talking about. Of all planetary aspects, this one seems to garner the most attention from readers, and it certainly generates the most mail. The reason for their interest is clear – this phenomenon is one of the few that affects everyone in a fairly uniform way, and its effects are always obvious. Once you begin to pay attention to how events in your life change during these phases, you will soon see how important it is to take note of them. Keeping track of Mercury retrograde periods can allow you to increase your productivity and avoid at least some of the frustration they can bring about. This API serves JSON over HTTPs. It allows you to determine whether Mercury is in retrograde for a given date.
📚 Documentation & Examples
Everything you need to integrate with Mercury Retrograde API
🚀 Quick Start Examples
// Mercury Retrograde API API Example
const response = await fetch('https://mercuryretrogradeapi.com/about.html', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
A Quick Guide to the Mercury Retrograde API
Are you tired of constantly experiencing disruptions in communication, plans, and technology during Mercury retrograde? Well, the Mercury Retrograde API can help you access the current phase of the dreaded retrograde cycle.
Here's a quick guide on how to use the API in JavaScript.
API Endpoints
Current Status Endpoint
This endpoint retrieves the current Mercury retrograde phase - either "retrograde", "direct", or "shadow":
https://mercuryretrogradeapi.com/api?date=today
Date Status Endpoint
This endpoint retrieves the Mercury retrograde phase on a specific date. Replace "YYYY/MM/DD" with the desired date:
https://mercuryretrogradeapi.com/api?date=YYYY/MM/DD
Example API Code
Fetch Request
To make API requests in JavaScript, we can use the fetch
method:
fetch("https://mercuryretrogradeapi.com/api?date=today")
.then(response => response.json())
.then(data => console.log(data.phase))
.catch(error => console.error(error));
Axios Request
Alternatively, we can use the axios
library to make requests:
axios.get("https://mercuryretrogradeapi.com/api?date=today")
.then(response => console.log(response.data.phase))
.catch(error => console.error(error));
jQuery Request
We can also use the $.get
method in jQuery:
$.get("https://mercuryretrogradeapi.com/api?date=today", function(data) {
console.log(data.phase);
});
Request a Specific Date
To retrieve the Mercury retrograde phase on a specific date, we can replace the date
parameter with the desired date:
fetch("https://mercuryretrogradeapi.com/api?date=2022/03/05")
.then(response => response.json())
.then(data => console.log(data.phase))
.catch(error => console.error(error));
Now you have the power to access the Mercury retrograde phase with just a few lines of JavaScript code. Happy coding!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes