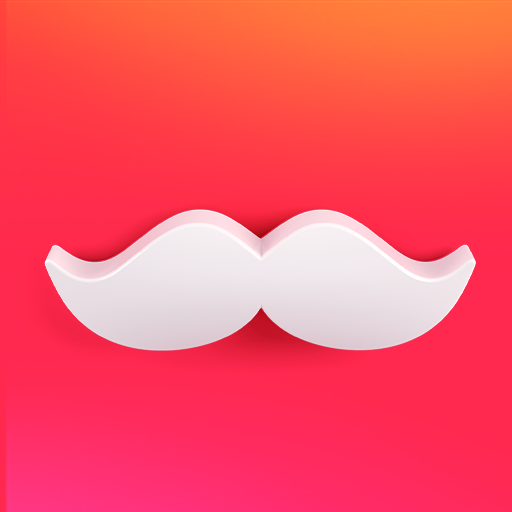
Rappi
ShoppingA Quick Guide to the Rappi Public API
If you're new to Rappi's public API, you might wonder what it is and how it can help you. Rappi is a popular on-demand delivery app that operates in various Latin American markets. The company offers a public API that lets third-party developers build apps and services that integrate with Rappi's platform.
In this article, we'll give you a quick rundown of the Rappi public API and show you some example code in JavaScript.
How to Get Started with the Rappi API
Before you can use the Rappi API, you'll need to sign up for a developer account and get an API key. Here's how to do it:
- Go to the Rappi Developer Portal.
- Click on the "Sign Up" button and create a new account.
- Once you're logged in, click on the "My Apps" link and create a new app.
- You'll receive an API key that you can use to authenticate your API requests.
Example API Calls with JavaScript
Now that you have an API key, you can start making API calls. Here are some example API calls you can make using JavaScript:
Get Stores
To get a list of stores available in your area, you can use the GET /stores
endpoint. Here's an example code snippet that shows how to make this API call using the axios library:
const axios = require('axios');
const url = 'https://api-lbs.rappi.com/api/v2/stores?latitude=4.710989&longitude=-74.072091';
const headers = {
'Content-Type': 'application/json',
'Authorization': '<your_api_key_here>'
};
axios.get(url, { headers })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
Get Products
To get a list of products available in a store, you can use the GET /stores/{store_id}/products
endpoint. Here's an example:
const axios = require('axios');
const url = 'https://api-lbs.rappi.com/api/v2/stores/12345/products';
const headers = {
'Content-Type': 'application/json',
'Authorization': '<your_api_key_here>'
};
axios.get(url, { headers })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
Create an Order
To create an order, you can use the POST /orders
endpoint. Here's an example:
const axios = require('axios');
const url = 'https://api-lbs.rappi.com/api/v2/orders';
const data = {
"store_id": "12345",
"latitude": "4.710989",
"longitude": "-74.072091",
"payment_data": {
"payment_type_id": "1",
"card_number": "1234567890123456",
"security_code": "123",
"expiration_month": "12",
"expiration_year": "22"
},
"order_items": [
{
"product_id": "54321",
"quantity": 2
}
]
};
const headers = {
'Content-Type': 'application/json',
'Authorization': '<your_api_key_here>'
};
axios.post(url, data, { headers })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
Final Thoughts
We hope this quick guide to the Rappi public API has been helpful to you. With the API, you can build innovative apps and services that enhance the customer experience and drive business growth. If you have any questions or need further assistance, be sure to check out the Rappi developer portal for more information and resources.