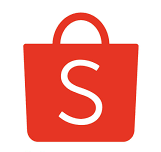
Shopee
ShoppingIntroduction to Shopee Open Platform API
Shopee Open Platform API is a public API provided by Shopee, an e-commerce platform based in Southeast Asia and Taiwan. The API allows developers to access Shopee’s platform resources to build ecommerce applications. This guide will provide an overview of the platform API and provide example codes in JavaScript.
Authentication
To use Shopee Open Platform API, you will need to register for a test or production account and generate an API key. With this key, you can access the API.
Examples of Shopee Open Platform API Codes in JavaScript
Get Shop Categories
const axios = require("axios");
const getShopCategories = async () => {
const config = {
headers: {
Authorization: "Bearer {access_token}",
"Content-Type": "application/json",
},
data: {
partner_id: {partner_id},
shopid: {shop_id},
timestamp: Math.floor(Date.now() / 1000),
},
};
await axios
.post("https://partner.test-stable.shopeemobile.com/api/v2/shop/get_categories", config)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error);
});
};
This code retrieves all of the shop categories of a Shopee seller’s shop.
Get Shop Orders
const axios = require("axios");
const getShopOrders = async () => {
const config = {
headers: {
Authorization: "Bearer {access_token}",
"Content-Type": "application/json",
},
data: {
partner_id: {partner_id},
shopid: {shop_id},
timestamp: Math.floor(Date.now() / 1000),
pagination_offset: 0,
pagination_entries_per_page: 50,
},
};
await axios
.post("https://partner.test-stable.shopeemobile.com/api/v2/orders/get", config)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error);
});
};
This code retrieves all of the orders of a Shopee seller’s shop.
Conclusion
Shopee Open Platform API is a powerful tool for developers to create ecommerce applications. In this guide, we’ve provided some example codes to help you get started. Feel free to explore the official documentation to better understand the capabilities of the API. Happy coding!