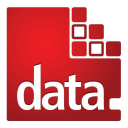
π Documentation & Examples
Everything you need to integrate with Australian Open Government
π Quick Start Examples
// Australian Open Government API Example
const response = await fetch('https://www.data.gov.au/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring API Docs for Data.gov.au
If you are looking for a comprehensive list of available open data sets in Australia, Data.gov.au is the place to be. It provides a publicly accessible API for retrieving datasets, resources, organizations, user accounts, and more.
Here's a brief overview of how to use the Data.gov.au API in JavaScript with examples of some of the most common requests:
Getting Started with Data.gov.au API
To use the API, you first need an API key, which you can obtain for free by creating an account on Data.gov.au. Once you have your API key, you can start making API requests.
The Data.gov.au API provides endpoints for various types of data, including datasets, resources, organizations, and user accounts. You can access these endpoints by making HTTP requests to the Data.gov.au API service using your API key.
Retrieving a Dataset
To retrieve a dataset, you can use the URL format https://data.gov.au/api/3/action/package_show?id=<dataset-id>
and perform an HTTP GET
request. Here's an example JavaScript code that demonstrates how to retrieve a dataset with the Data.gov.au API.
const datasetId = "australian-sensor-network-experiment";
const apiKey = "<your-api-key>";
fetch(`https://data.gov.au/api/3/action/package_show?id=${datasetId}`, {
headers: {
Authorization: apiKey,
},
})
.then((res) => res.json())
.then((data) => console.log(data));
Example Result
{
help: "",
success: true,
result: {
metadata_created: "2015-08-04T01:47:08.875310",
name: "australian-sensor-network-experiment",
title: "Australian Sensor Network Experiment",
...
}
}
Retrieving a Resource
To retrieve a resource from a dataset, you can use the URL format https://data.gov.au/api/3/action/resource_show?id=<resource-id>
and perform an HTTP GET
request. Here's an example JavaScript code that demonstrates how to retrieve a resource with the Data.gov.au API.
const resourceId = "00023e23-90e2-4ae8-b7d7-ddfebc2c66f0";
const apiKey = "<your-api-key>";
fetch(`https://data.gov.au/api/3/action/resource_show?id=${resourceId}`, {
headers: {
Authorization: apiKey,
},
})
.then((res) => res.json())
.then((data) => console.log(data));
Example Result
{
help: "",
success: true,
result: {
cache_last_updated: null,
cache_url: null,
created: "2020-07-17T13:38:55.858614",
datastore_active: false,
...
}
}
Retrieving Organizations
To retrieve a list of organizations, you can use the URL format https://data.gov.au/api/3/action/organization_list
and perform an HTTP GET
request. Here's an example JavaScript code that demonstrates how to retrieve organizations with the Data.gov.au API.
const apiKey = "<your-api-key>";
fetch(`https://data.gov.au/api/3/action/organization_list`, {
headers: {
Authorization: apiKey,
},
})
.then((res) => res.json())
.then((data) => console.log(data));
Example Result
{
help: "",
success: true,
result: [
"abc",
"def",
...
]
}
Retrieving User Accounts
To retrieve a list of user accounts, you can use the URL format https://data.gov.au/api/3/action/user_list
and perform an HTTP GET
request. Here's an example JavaScript code that demonstrates how to retrieve user accounts with the Data.gov.au API.
const apiKey = "<your-api-key>";
fetch(`https://data.gov.au/api/3/action/user_list`, {
headers: {
Authorization: apiKey,
},
})
.then((res) => res.json())
.then((data) => console.log(data));
Example Result
{
help: "",
success: true,
result: [
{
apikey: "<user-api-key>",
created: "2021-07-01T12:30:00",
email_hash: "123456789abcdefg",
fullname: "John Doe",
...
},
...
]
}
Conclusion
Data.gov.au provides a comprehensive API for accessing publicly available open data sets. With this API, you can query datasets, resources, organizations, and user accounts, and retrieve data in JSON format. This makes it easy for developers to build applications that leverage Australian government data.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes