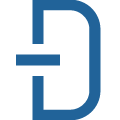
United State Open Government
Data AccessExploring Public Data with Data.gov’s API
Data.gov is the central repository for all U.S. Government datasets. It’s an excellent resource for government agencies, developers, and researchers to access publicly available data that can help with analysis, decision-making, and problem-solving.
To access Data.gov’s vast data collection, you can use their Application Programming Interface (API). An API is a way for programs to interact with each other. By using Data.gov’s API, you can access raw data that is open, free, and available to everyone.
Let’s dive into some examples of how to use Data.gov’s API with JavaScript!
Prerequisites
Before you start using Data.gov’s API, you’ll need an API key. You can sign up for a free API key on the Data.gov developer page.
After signing up, you will receive an email with your API key. Keep this key safe as it will be needed in all API requests.
Example 1: Getting a List of Datasets
To get started, let’s retrieve a list of available datasets from Data.gov. Here’s the JavaScript code to do so:
const apiUrl = "https://api.gov/data/v1/datasets";
const apiKey = "<YOUR_API_KEY>";
fetch(`${apiUrl}/metadata?api_key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are using the fetch
method to make an HTTP GET request to Data.gov’s API endpoint. We are also passing our API key as a query parameter in the URL.
In the .then
method, we’re converting the response to JSON format and logging it to the console. In the .catch
method, we’re logging any errors to the console.
Example 2: Searching for a Specific Dataset
Now, let’s see how to search for a specific dataset using Data.gov’s API. Here’s the code:
const apiUrl = "https://api.gov/data/v1/datasets";
const apiKey = "<YOUR_API_KEY>";
const searchTerm = "covid-19";
fetch(`${apiUrl}/metadata?api_key=${apiKey}&q=${searchTerm}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we’ve added a searchTerm
variable, which we pass as a query parameter in the URL. This tells Data.gov to return only datasets that match our search term.
Example 3: Filtering Results
Finally, let’s explore how to filter our search results. Here’s how:
const apiUrl = "https://api.gov/data/v1/datasets";
const apiKey = "<YOUR_API_KEY>";
const searchTerm = "covid-19";
const filters = "tags=health";
fetch(`${apiUrl}/metadata?api_key=${apiKey}&q=${searchTerm}&${filters}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we’ve added a filters
variable to narrow down our results based on specific tags. You can add multiple filters by separating them with an ampersand (&
).
Conclusion
Data.gov’s API is a powerful tool for accessing publicly available data. By using JavaScript, you can easily retrieve, filter, and analyze datasets to gain insights and make informed decisions.
We hope these examples helped you get started with Data.gov’s API and JavaScript. Try it out for yourself and see what you can discover!