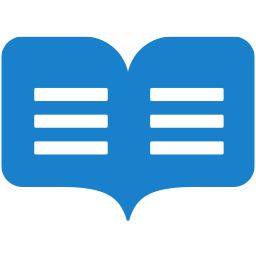
Intercom API
AutomationDiscover all the incredible capabilities of our platform so that you can build context-relevant, action-oriented apps directly on top of Intercom with ease - whether you're publicly integrating your service with ours, or you're building for your own team's private usage.
π Documentation & Examples
Everything you need to integrate with Intercom API
π Quick Start Examples
// Intercom API API Example
const response = await fetch('https://developers.intercom.com/building-apps/docs', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Introduction to Intercom Public API
Intercom offers a public API that allows developers to fetch data from their application programmatically. This data can be used to integrate with other services or to build custom applications that leverage Intercom data.
Intercom provides a number of API endpoints that can be used to fetch data related to users, conversations, message threads, and more. In this blog post, we will explore some of the most popular API endpoints offered by Intercom and provide JavaScript example code for each.
Authenticating with Intercom API
Before you start using the Intercom API, you need to generate an access token for your application. You can do this by logging into your Intercom account and generating a "Personal Access Token" from the API key settings.
Once you have generated your access token, you can use it to authenticate your requests by including it in the "Authorization" header as follows:
const baseURL = "https://api.intercom.io";
const accessToken = "YOUR_ACCESS_TOKEN";
axios.defaults.headers.common.Authorization = `Bearer ${accessToken}`;
axios.defaults.headers.post["Content-Type"] = "application/json";
User API Endpoints
The User API endpoints are used to fetch information about the users in your Intercom account. You can use these endpoints to fetch user attributes, such as name, email address, and user ID.
List All Users in Your Account
axios.get(`${baseURL}/users`, {
params: {
per_page: 50
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
List User by ID
axios.get(`${baseURL}/users/${userId}`)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Create a New User
axios.post(`${baseURL}/users`, {
email: "john.doe@example.com",
name: "John Doe",
signed_up_at: new Date().toISOString()
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Conversation API Endpoints
The Conversation API endpoints are used to fetch information about the conversations in your Intercom account.
List All Conversations
axios.get(`${baseURL}/conversations`, {
params: {
per_page: 50
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
List Conversation by ID
axios.get(`${baseURL}/conversations/${conversationId}`)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Create a New Conversation
axios.post(`${baseURL}/conversations`, {
message_type: "inapp",
body: "Hello, how can I assist you?",
from: {
type: "admin",
id: "YOUR_ADMIN_ID"
},
to: {
type: "user",
id: "USER_ID"
},
assignee: {
type: "admin",
id: "YOUR_ADMIN_ID"
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Summary
Intercom's public API offers a wide range of endpoints and functionalities that enable developers to programmatically access and manage data within their Intercom account. In this blog post, we have provided some example JavaScript code for some of the most popular API endpoints.
Remember to always specify your API access token to authenticate your requests, and consult Intercom's API documentation for additional information and functionality.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes