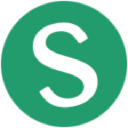
Sejda PDF API
AutomationSimplify PDF Processing with Sejda's Public API
Sejda's public API provides developers with a simple way to integrate PDF processing features into their web or mobile applications. With this API, developers can access various PDF manipulation features, including merging, splitting, converting, and editing PDFs, and perform them programmatically.
Getting Started with Sejda's Public API
Before we dive into using the Sejda API, let's discuss the API's requirements.
First, developers need to sign up for an API key by visiting https://www.sejda.com/developers. The API key is necessary for all API requests, and developers are advised to keep it secure to prevent unauthorized access to their account.
Once you have your API key, you can use it to make a test API request like so:
fetch("https://api.sejda.com/v1/tasks", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": "Token MY_API_KEY"
},
body: JSON.stringify({
"tasks": [
{
"name": "merge",
"files": [
{
"url": "http://url.to/my/first.pdf"
},
{
"url": "http://url.to/my/second.pdf"
}
]
}
]
})
})
.then(res => res.json())
.then(response => console.log(response.task.id))
.catch(error => console.error("Error: " + error));
The above JavaScript code sends a POST request with a sample merge task that includes two sample PDF files. The API returns a JSON response that includes the task ID, which can be used to check the task's status.
The Authorization
header in the request contains your API key.
PDF Processing with Sejda's Public API in JavaScript
Now that you have made your first API request let's explore the various PDF processing features provided by the Sejda API through JavaScript examples.
Merging PDFs
The Sejda API allows you to merge multiple PDF files into one using the merge
task. Here is an example code:
fetch("https://api.sejda.com/v1/tasks", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": "Token MY_API_KEY"
},
body: JSON.stringify({
"tasks": [
{
"name": "merge",
"files": [
{
"url": "http://url.to/my/first.pdf"
},
{
"url": "http://url.to/my/second.pdf"
}
]
}
]
})
})
.then(res => res.json())
.then(response => {
if (response.status === "SUCCESS") {
// The merged PDF file URL is in response.outputFiles[0].url
console.log("Merged file URL: ", response.outputFiles[0].url);
} else {
console.error(response.status);
}
})
.catch(error => console.error("Error: " + error));
Splitting PDFs
The API also allows you to split a PDF file into multiple files using the split
task. Here is an example code:
fetch("https://api.sejda.com/v1/tasks", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": "Token MY_API_KEY"
},
body: JSON.stringify({
"tasks": [
{
"name": "split",
"files": [
{
"url": "http://url.to/my/large.pdf"
}
],
"splitBy": "pages",
"splitMode": "specific",
"specificRanges": [
{
"pages": "1-5, 10"
},
{
"pages": "6-9"
}
]
}
]
})
})
.then(res => res.json())
.then(response => {
if (response.status === "SUCCESS") {
// The split PDF file URLs are in response.outputFiles
console.log("Split file URLs: ", response.outputFiles.map(f => f.url));
} else {
console.error(response.status);
}
})
.catch(error => console.error("Error: " + error));
Extracting Pages
The API also provides an easy way to extract pages from a PDF file. Here is an example code:
fetch("https://api.sejda.com/v1/tasks", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": "Token MY_API_KEY"
},
body: JSON.stringify({
"tasks": [
{
"name": "extractPages",
"files": [
{
"url": "http://url.to/my/large.pdf"
}
],
"extractPages": "1-5, 10"
}
]
})
})
.then(res => res.json())
.then(response => {
if (response.status === "SUCCESS") {
// The extracted PDF file URL is in response.outputFiles[0].url
console.log("Extracted file URL: ", response.outputFiles[0].url);
} else {
console.error(response.status);
}
})
.catch(error => console.error("Error: " + error));
Converting PDFs
Finally, the Sejda API also allows developers to convert PDFs to other file formats, including images, HTML, and Microsoft Office formats. Here is an example code to convert a PDF to an image:
fetch("https://api.sejda.com/v1/tasks", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": "Token MY_API_KEY"
},
body: JSON.stringify({
"tasks": [
{
"name": "pdfToImg",
"files": [
{
"url": "http://url.to/my/pdf/document.pdf"
}
],
"format": "PNG",
"resolution": 300,
"colorSpace": "RGB"
}
]
})
})
.then(res => res.json())
.then(response => {
if (response.status === "SUCCESS") {
// The converted image file URL is in response.outputFiles[0].url
console.log("Converted image URL: ", response.outputFiles[0].url);
} else {
console.error(response.status);
}
})
.catch(error => console.error("Error: " + error));
Conclusion
In conclusion, Sejda's public API provides developers with a powerful and easy-to-use tool for PDF processing. With a few lines of JavaScript code, developers can integrate various PDF manipulation features into their applications with ease. These features include merging, splitting, extracting pages, and converting PDFs. Don't forget to sign up for the API key, and always follow the API's best practices and guidelines to avoid any issues.