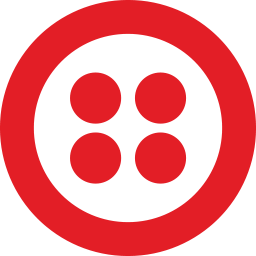
Twilio API
AutomationUsing Twilio API for Messaging and Calls
Are you looking for ways to integrate messaging or calling functionality in your web or mobile app? Twilio offers an API that makes it easy to build communication solutions. This blog will give you a quick overview of the Twilio API and share some example code snippets in JavaScript to get you started.
Twilio API
Twilio is a cloud-based platform that enables you to send and receive SMS, MMS, and voice messages using APIs. It offers a rich set of features to help you build scalable and reliable communication solutions. The Twilio API can be accessed using HTTPS requests and is designed to be developer-friendly with clear documentation and helper libraries.
Getting Started
- Sign up for a Twilio account
- Create your first Twilio phone number
- Install Twilio helper library for Node.js
// Install Twilio library using npm
npm install twilio
- Get Twilio credentials and configure client
// Require the Twilio module and create a client
const accountSid = 'ACxxxxxxxxxxxxxxxxxxxxxxxxxxxxx';
const authToken = 'your_auth_token';
const client = require('twilio')(accountSid, authToken);
Sending SMS
Sending a text message using Twilio API requires you to have a Twilio phone number and the recipient's phone number. Here is a simple example code that sends an SMS message using Twilio API.
// Send an SMS message
client.messages
.create({
body: 'Hello from Twilio API',
from: '+1xxxxxxxxxx', // Twilio phone number
to: '+1yyyyyyyyyy' // Recipient phone number
})
.then(message => console.log(message.sid))
.catch(error => console.log(error));
Making Calls
Twilio API also allows you to make phone calls programmatically. To make a call, you need to provide a TwiML (Twilio Markup Language) script that instructs the call on what to do. Here is an example code that makes a call and plays a message using a TwiML script.
// Make a call using a TwiML script
client.calls
.create({
url: 'http://demo.twilio.com/docs/voice.xml', // TwiML script URL
to: '+1yyyyyyyyyy', // Recipient phone number
from: '+1xxxxxxxxxx' // Twilio phone number
})
.then(call => console.log(call.sid))
.catch(error => console.log(error));
Conclusion
Using Twilio API, you can quickly and easily add messaging and calling functionality to your web or mobile app. Twilio provides a rich set of APIs and SDKs along with detailed documentation and community support that makes it developer-friendly. I hope this article gave you a quick overview and some example code to get started with the Twilio API.