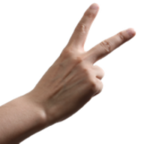
ObjectCut
AutomationIntroduction to ObjectCut Public API
ObjectCut Public API is designed to allow developers to integrate image background removal capabilities into their applications. With this API, developers can quickly remove the background of an image and focus only on the object/image in the foreground. In this blog post, we will explore how to use the ObjectCut Public API with JavaScript, using example code.
Getting started with the ObjectCut Public API
Before we dive into using the ObjectCut Public API with JavaScript, let us first understand how to get started with the API. Firstly, you need to sign up for the API and get an API Key. The API Key will be used to authenticate your requests to the API.
To sign up for the ObjectCut Public API, visit the website https://objectcut.com and follow the registration process. Once you have signed up for the API, you will receive an API Key that you can use to make requests to the API.
Using the ObjectCut Public API with JavaScript
To use the ObjectCut Public API with JavaScript, we will use the fetch()
method to make requests to the API.
Here's an example code to use the fetch()
method with the ObjectCut Public API to remove the background of an image:
// set the API endpoint URL
const apiUrl = "https://api.objectcut.com/v1/remove-background";
// set your API Key
const apiKey = "your-api-key-here";
// set the image URL you want to remove the background from
const imageUrl = "https://example.com/image.jpg";
// create a FormData object to send data in the request body
const formData = new FormData();
formData.append("image_url", imageUrl);
// make a request to the API
fetch(apiUrl, {
method: "POST",
headers: {
"Api-Key": apiKey
},
body: formData
})
.then(response => response.json())
.then(data => {
// handle the API response
console.log(data);
})
.catch(error => {
// handle errors
console.error(error);
});
In the above code, we have set the API endpoint URL, the API Key, and the image URL that we want to remove the background from. We create a FormData
object to send the data in the request body. We then make a fetch()
request to the API, passing the API Key in the request headers, and the FormData object in the request body. Finally, we handle the API response in the then()
block and handle errors in the catch()
block.
Conclusion
In this blog post, we explored how to use the ObjectCut Public API with JavaScript, with the help of example code. With the ObjectCut Public API, developers can easily remove the background of an image and focus on the object in the foreground. Try using the API with your JavaScript application and see the results for yourself.