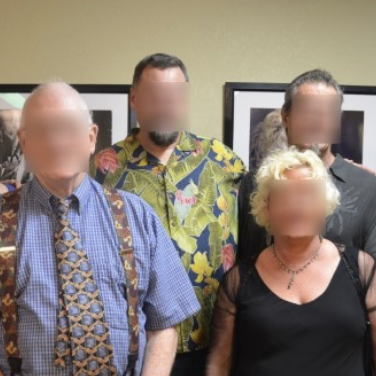
PixLab
AutomationDiscovering PixLab API
If you're a developer looking for an image and video analysis tool to integrate with your code, PixLab API might be a good choice for you. With PixLab API, you can analyze, manipulate, and tag your images and videos in different programming languages.
Getting Started with PixLab API
Before getting started with PixLab API, you need to sign up for an account to obtain your API key. The API key is the unique identifier to access the API. Once you have that, you can start using the API by calling one of the endpoints with your API key.
Here are some examples of how to make a request using PixLab API in JavaScript:
// Import the required libraries.
const fetch = require("node-fetch");
// Set the endpoint URL and parameters.
const url = "https://api.pixlab.io/blur";
const apiKey = "YOUR_API_KEY";
const imgURL = "https://example.com/image.jpg";
const radius = 10;
// Set the headers to include your API key.
const headers = {"Content-Type": "application/json", "key": apiKey};
// Make the request using fetch.
fetch(`${url}?img=${imgURL}&radius=${radius}`, { headers: headers })
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error(err));
In this example, we use the node-fetch
library to make a request to the blur
endpoint with a given image URL and a blur radius. The response will be returned in JSON format.
For more information about how to use the different endpoints available in PixLab API, consult their official documentation.
Conclusion
PixLab API is a powerful tool that can help you analyze and manipulate your images and videos in different programming languages. With the provided examples, you should be able to quickly start using the API. Happy coding!