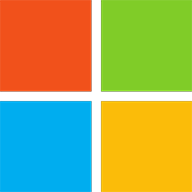
OneDrive
Cloud Storage & File SharingIntroduction to OneDrive API
OneDrive is a file hosting and synchronization service from Microsoft that allows users to store and share their data in the cloud. OneDrive API provides developers with a set of tools and techniques to build web or mobile applications that can interact with OneDrive data. In this article, we will discuss how to use OneDrive API in JavaScript.
Getting Started with OneDrive API
Before you can use the OneDrive API, you need to register your application with Microsoft and obtain an access token. Follow the steps below to get started with OneDrive API:
- Go to https://apps.dev.microsoft.com and sign in with your Microsoft account.
- Click on Add an app and fill in the required details.
- Click on Add Platform and select Web.
- Enter the Redirect URL for your application. This is the URL where Microsoft will send the access token after the user has granted permission.
- Click on Generate New Password to generate a new password for your app.
- Note down the Application ID and Application Secret. You will need these later.
- Go to https://login.microsoftonline.com/common/oauth2/v2.0/authorize and replace YOUR_APP_ID and YOUR_REDIRECT_URI with your actual values.
- Sign in with your Microsoft account and grant permission to your app. Microsoft will redirect you to your Redirect URL with a code parameter in the query string.
You can now exchange this code for an access token by making a POST request to https://login.microsoftonline.com/common/oauth2/v2.0/token. Here is an example code snippet that does this in JavaScript:
const request = new XMLHttpRequest();
request.open('POST', 'https://login.microsoftonline.com/common/oauth2/v2.0/token');
request.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
const data = `client_id=${encodeURIComponent(YOUR_APP_ID)}`
+ `&scope=${encodeURIComponent('files.readwrite.all offline_access')}`
+ `&code=${encodeURIComponent(AUTHORIZATION_CODE)}`
+ `&redirect_uri=${encodeURIComponent('YOUR_REDIRECT_URI')}`
+ `&grant_type=authorization_code`
+ `&client_secret=${encodeURIComponent(YOUR_APP_SECRET)}`;
request.send(data);
request.onreadystatechange = function () {
if (this.readyState === 4 && this.status === 200) {
const response = JSON.parse(this.responseText);
const access_token = response.access_token;
const refresh_token = response.refresh_token;
const expires_in = response.expires_in;
}
};
Examples of Using OneDrive API
Once you have obtained an access token, you can use OneDrive API methods to work with files and folders stored on OneDrive. Here are some examples of using OneDrive API:
List All Files and Folders
This example demonstrates how to list all files and folders stored on OneDrive.
const request = new XMLHttpRequest();
request.open('GET', 'https://graph.microsoft.com/v1.0/me/drive/root/children');
request.setRequestHeader('Authorization', `Bearer ${YOUR_ACCESS_TOKEN}`);
request.send();
request.onreadystatechange = function () {
if (this.readyState === 4 && this.status === 200) {
const response = JSON.parse(this.responseText);
const files = response.value;
}
};
Upload a File
This example demonstrates how to upload a file to OneDrive.
const fileInput = document.getElementById('file-input');
const file = fileInput.files[0];
const reader = new FileReader();
reader.readAsArrayBuffer(file);
reader.onloadend = function () {
const request = new XMLHttpRequest();
request.open('PUT', `https://graph.microsoft.com/v1.0/me/drive/root:/${encodeURIComponent(file.name)}:/content`);
request.setRequestHeader('Authorization', `Bearer ${YOUR_ACCESS_TOKEN}`);
request.setRequestHeader('Content-Type', file.type);
request.send(reader.result);
request.onreadystatechange = function () {
if (this.readyState === 4 && this.status === 200) {
console.log('File uploaded successfully.');
}
};
};
Download a File
This example demonstrates how to download a file from OneDrive.
const request = new XMLHttpRequest();
request.open('GET', `https://graph.microsoft.com/v1.0/me/drive/items/${encodeURIComponent(FILE_ID)}/content`);
request.setRequestHeader('Authorization', `Bearer ${YOUR_ACCESS_TOKEN}`);
request.responseType = 'arraybuffer';
request.send();
request.onreadystatechange = function () {
if (this.readyState === 4 && this.status === 200) {
const blob = new Blob([this.response]);
const link = document.createElement('a');
link.href = URL.createObjectURL(blob);
link.download = FILE_NAME;
link.click();
}
};
Conclusion
OneDrive API is a powerful tool that can be used to build cloud-based applications that are able to interact with OneDrive data. In this article, we have discussed how to use OneDrive API in JavaScript and provided some examples of how to work with files and folders stored on OneDrive. We hope that this article has been helpful in enabling you to develop your own OneDrive-powered applications.