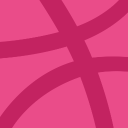
Dribble
MediaA Beginner's Guide to the Dribbble API in JavaScript
Are you a developer looking to integrate Dribbble's features into your web application? Then look no further than the Dribbble API, which allows you to access a wealth of data on the world's premier online community for designers.
In this guide, we will walk you through all you need to know to get started with the Dribbble API in JavaScript, no matter your level of expertise.
Setting Up Your Environment
First things first, you'll need to have a few things in place before you can start making requests to the Dribbble API.
Register for an API Key
In order to access the Dribbble API, you will need to register for an API key on their developer website. You can find the registration page here: http://developer.dribbble.com/v1/.
Install an HTTP Library
Next, you'll need to install an HTTP library that can handle requests to the Dribbble API. There are several options out there, but we recommend using Axios, a popular HTTP client for JavaScript. You can install it using npm
or yarn
.
npm install axios
Import Axios
In your JavaScript file, import the Axios library so you can start making requests to the Dribbble API. Below is an example of how to do this:
import axios from 'axios';
Making API Requests
Once your environment is set up, you can start making requests to the Dribbble API. Below are some examples of how to do this using Axios.
Get Shots
To get a list of shots from the Dribbble API, use the following code:
axios.get('https://api.dribbble.com/v1/shots', {
params: {
access_token: '{YOUR_ACCESS_TOKEN}'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Note that you'll need to replace {YOUR_ACCESS_TOKEN}
with your personal access token, which you can find in your developer account on the Dribbble website.
Get a Specific Shot
To get details on a specific shot, use the following code:
axios.get('https://api.dribbble.com/v1/shots/12345', {
params: {
access_token: '{YOUR_ACCESS_TOKEN}'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Again, replace 12345
with the ID of the shot you want to retrieve.
Get User Info
To retrieve information on a Dribbble user, use the following code:
axios.get('https://api.dribbble.com/v1/users/{USERNAME}', {
params: {
access_token: '{YOUR_ACCESS_TOKEN}'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Replace {USERNAME}
with the Dribbble username you want to retrieve information on.
Wrapping Up
There you have it! A beginner's guide to the Dribbble API in JavaScript. Hopefully, this guide has given you a good starting point for working with the Dribbble API and has opened up a whole new world of possibilities for your web applications. Happy coding!