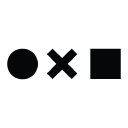
Noun Project
MediaUse the Noun Project Icon API to tell visual stories, create real-time infographics, build interactive games, or whatever crazy idea you have. With our API you can bring a growing collection of high quality symbols to anything you create. Innovators are using the Noun Project API to tell visual stories, educate children with autism, create real-time infographics, build interactive games, and personalize 3-D printed products. The possibilities are endless and we can't wait to see what you create!
📚 Documentation & Examples
Everything you need to integrate with Noun Project
🚀 Quick Start Examples
// Noun Project API Example
const response = await fetch('http://api.thenounproject.com/index.html', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring the Public API Docs of The Noun Project
The Noun Project is a popular platform that offers a vast collection of icons and symbols for designers, developers, and content creators. And the great news is that they also provide a public API that we can use to access their catalog of icons and integrate them into our own applications.
In this blog post, we will explore The Noun Project's public API documentation and demonstrate some example code snippets in JavaScript to help you get started with using this API.
Getting Started
To use The Noun Project's API, we need to first sign up and obtain an API key. We can sign up for free from their website and generate our API token. Once we have the API key, we can start exploring the documentation and building our own applications.
Documentation Overview
The Noun Project's API documentation is simple and well-organized. The main endpoints are logically separated, and each endpoint provides a detailed description of its purpose, parameters, and response format.
The endpoints are categorized based on the different types of requests we can make, like icons, collections, users, and more. Each endpoint provides example requests and responses, which is helpful in quickly understanding how to access data and what data will be returned.
Example Code in JavaScript
Now that we have a basic understanding of how the API works and its documentation structure, let's jump into some sample code snippets in JavaScript.
Retrieving Icons
We can retrieve a list of icons by sending an HTTP GET request to the https://api.thenounproject.com
endpoint with the path /icons
. We can also provide query parameters to filter and page through the results.
const axios = require('axios');
const API_TOKEN = 'your-token-here';
const API_URL = 'https://api.thenounproject.com/v2/';
const fetchIcons = async (params) => {
try {
const { data } = await axios.get(`${API_URL}icons`, {
params,
headers: {
'X-ApiKey': API_TOKEN,
},
});
return data;
} catch (error) {
console.error(error);
}
};
fetchIcons({ term: 'cat', limit: 10 }).then((data) => {
console.log(data);
});
In the above example, we use the axios
library to send an HTTP GET request to the API endpoint. We provide the API token in the header, and pass the query parameters for filtering (search term) and limiting (number of results). The fetchIcons
function returns a promise that will resolve with the response data.
Retrieving a Single Icon
We can retrieve a specific icon by sending an HTTP GET request to the https://api.thenounproject.com
endpoint with the path /icons/{icon_id}
. We need to replace the {icon_id}
placeholder with the actual icon ID.
const fetchIcon = async (iconId) => {
try {
const { data } = await axios.get(`${API_URL}icons/${iconId}`, {
headers: {
'X-ApiKey': API_TOKEN,
},
});
return data;
} catch (error) {
console.error(error);
}
};
fetchIcon('1234').then((data) => {
console.log(data);
});
In the above example, we use the fetchIcon
function to retrieve a specific icon by providing the icon ID as a parameter. The function sends an HTTP GET request to the API endpoint with the icon ID, and returns a promise that will resolve with the response data.
Conclusion
The Noun Project's public API is a powerful tool that can be used to access and integrate their icon collection into our own applications. The API documentation is clear and detailed, which makes it easy to understand and use.
In this blog post, we explored the API documentation and demonstrated some example code snippets in JavaScript to retrieve icons and a single icon. We hope that this blog post has been helpful in getting you started with using The Noun Project's public API.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes