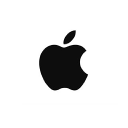
iTunes Search
MediaThe Search API allows you to place search fields in your website to search for content within the iTunes Store and Apple Books Store. You can search for a variety of content; including books, movies, podcasts, music, music videos, audiobooks, and TV shows. You can also call an ID-based lookup request to create mappings between your content library and the digital catalog.
📚 Documentation & Examples
Everything you need to integrate with iTunes Search
🚀 Quick Start Examples
// iTunes Search API Example
const response = await fetch('https://affiliate.itunes.apple.com/resources/documentation/itunes-store-web-service-search-api/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using the iTunes Search API
The iTunes Search API allows developers to search the iTunes Store's catalog of music, movies, TV shows, podcasts, and audiobooks. In this blog post, we'll cover the basics of using the iTunes Search API with JavaScript.
Getting Started
To start using the iTunes Search API, you'll need to create an affiliate account with Apple. Once you have an account, you'll need to generate an affiliate token, which you'll use in your API requests.
API Requests
API requests are made using HTTP GET requests to the following endpoint:
https://itunes.apple.com/search
The API takes several parameters in the query string to allow you to filter your search results. Here are some of the most common parameters:
term
: The search term or keyword(s) you want to search for.media
: The type of media you want to search for (e.g.music
,movie
,tvShow
,podcast
,audiobook
).entity
: The type of entity you want to search for (e.g.album
,song
,movie
,tvSeason
,podcast
,audiobook
).attribute
: The attribute you want to search for (e.g.actorTerm
,genreIndex
,languageTerm
).limit
: The maximum number of results to return.callback
: The name of the JavaScript function to be used as the callback when returning JSONP results.
Example Code
Here's an example of how to use the iTunes Search API with JavaScript:
function searchiTunes(term) {
const url = `https://itunes.apple.com/search?term=${term}&media=music&entity=song`;
fetch(url)
.then(response => response.json())
.then(data => {
// Handle the response data here
console.log(data);
})
.catch(error => {
// Handle the error here
console.error(error);
});
}
searchiTunes('Taylor Swift');
This code searches for Taylor Swift songs on iTunes and returns the results as JSON data. You can replace music
with any other media type and song
with any other entity type to search for other types of content.
Conclusion
The iTunes Search API is a powerful tool for developers to search the iTunes Store's catalog. With JavaScript, you can integrate the API into your web applications and start searching for music, movies, TV shows, podcasts, and audiobooks.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes