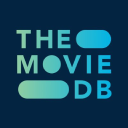
MovieDB
MediaExploring The Movie Database API
Are you interested in building an application that fetches movie information? Well, The Movie Database API provides a wealth of information on movies, TV series, and more. In this blog, we will explore how to use The Movie Database API with JavaScript.
API Documentation
The Movie Database API documentation can be found at https://www.themoviedb.org/documentation/api. You will first need to create an account to get an API key to use their services. Once you have your API key, you can start accessing the data.
API Endpoints
The API endpoints we will be covering in this blog include:
- Search Movies: This endpoint returns a list of movies that match your search query.
- Movie Details: This endpoint returns the full details of a movie.
- Movie Credits: This endpoint returns the credits (cast and crew) of a movie.
Example Code
To get started, let's create a JavaScript function that searches for a movie and returns a list of results.
async function searchMovie(query) {
const api_key = 'YOUR_API_KEY';
const url = `https://api.themoviedb.org/3/search/movie?api_key=${api_key}&query=${query}`;
const response = await fetch(url);
const data = await response.json();
return data.results;
}
In the above code, we are using the fetch
method to make a GET request to The Movie Database API endpoint. We are passing in our api_key
and the query
parameter that we want to search for. Once we get a response, we are converting it to JSON format and returning the list of results.
Let's say we want to view the details of a movie. We can create another JavaScript function that uses the movie's ID to fetch the details of the movie.
async function getMovieDetails(movieId) {
const api_key = 'YOUR_API_KEY';
const url = `https://api.themoviedb.org/3/movie/${movieId}?api_key=${api_key}`;
const response = await fetch(url);
const data = await response.json();
return data;
}
In this code, we are using the movieId
parameter to make a GET request to the Movie Details endpoint. Once we get a response, we are returning the data in JSON format.
Lastly, let's create a JavaScript function that fetches the credits of a movie using the movie's ID.
async function getMovieCredits(movieId) {
const api_key = 'YOUR_API_KEY';
const url = `https://api.themoviedb.org/3/movie/${movieId}/credits?api_key=${api_key}`;
const response = await fetch(url);
const data = await response.json();
return data.cast;
}
Here, we are using the movieId
parameter to make a GET request to the Movie Credits endpoint. Once we get a response, we are returning the cast details in JSON format.
That's it! With these functions, you can get started on building your movie application. You can combine these functions to search for a movie and get its details and credits.
Happy coding!