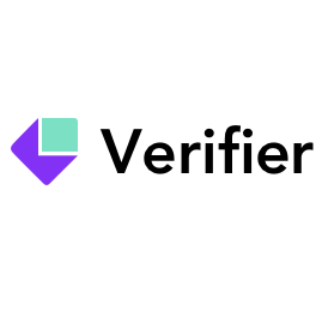
Email Verifier
DevelopmentHow to Verify Email Addresses using the email verifier API
If you are building an application that requires verifying email addresses, you can use the email verifier API to quickly and easily validate email addresses in real-time. In this tutorial, we will walk you through how to use this API with JavaScript.
Prerequisites
To follow along with this tutorial, you will need:
- A text editor
- A modern web browser
- Basic understanding of JavaScript programming language
Getting Started with the Email Verifier API
- Sign-up for an account with email validator API service.
- Obtain an API key.
- Choose an HTTP client to make requests to the API. We will be using
fetch
in this tutorial.
Example code:
const apiKey = 'YOUR_API_KEY'
const email = 'john@example.com'
fetch(`https://api.verifier.meetchopra.com/verify?email=${email}&key=${apiKey}`)
.then(response => response.json())
.then(data => {
console.log(data)
// Handle the response data here
})
.catch(error => console.error(error))
In this example, we have initialized the API key and email that we want to verify. We then use fetch
to send a GET request to the email verifier API endpoint with the email
and key
parameters.
When the API responds, we convert the response from JSON to JavaScript object using response.json()
, and then we log the data to the console.
The API returns a JSON response that looks something like:
{
"format_valid": true,
"smtp_check": true,
"catch_all": null,
"role": false,
"disposable": false,
"free": false,
"score": 0.8,
"success": true
}
It includes various details about the email address such as whether it is in the correct format, if it exists, is a disposable email etc.
Conclusion
That's it! In this tutorial, we showed you how to use the email verifier API to validate email addresses in real-time with JavaScript. You can use these concepts as a starting point to build out more complex email validation features for your application.