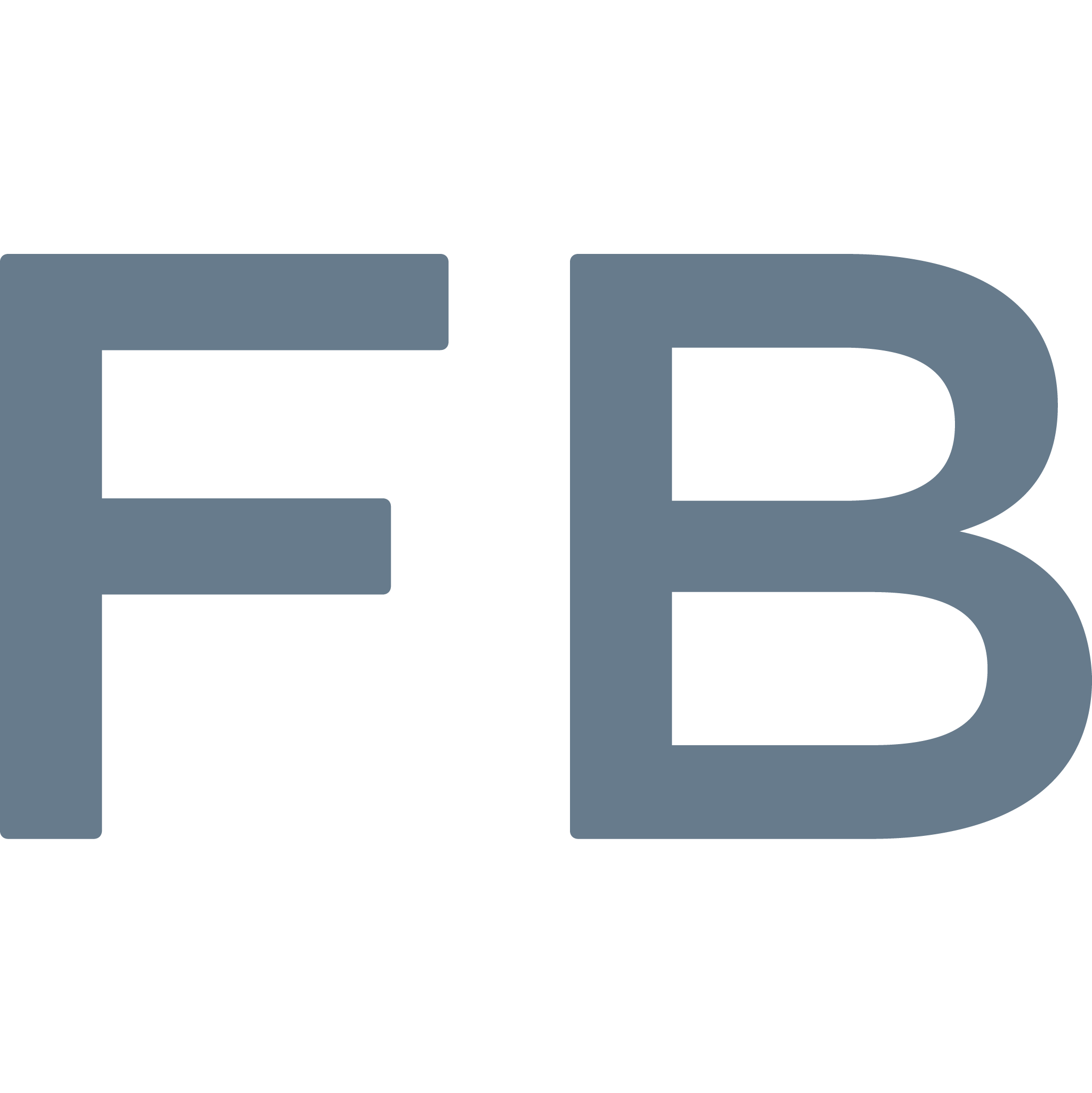
Facebook Marketing API
MarketingA brief overview of the Facebook Marketing API
The Facebook Marketing API is a powerful tool for developers, marketers and businesses to manage Facebook advertising campaigns and integrate with other Facebook products. The API allows developers to access Facebook's advertising features, such as creating campaigns, managing ads, and retrieving performance insights, among other things.
To get started with the Facebook Marketing API, visit the official documentation website at https://developers.facebook.com/docs/marketing-apis. Here, you will find a wealth of resources including:
- A comprehensive overview of the API, including its capabilities, requirements and guidelines.
- Guides and tutorials on how to use the API to perform specific actions such as creating ad campaigns, targeting specific audiences and retrieving insights.
- Reference documentation that provides detailed explanations of each API endpoint, including the parameters and responses.
- Sample code snippets for various programming languages, including JavaScript, PHP, Python and Ruby.
In this article, we will be focusing on JavaScript examples of how to use the Facebook Marketing API to perform common actions.
Authentication
One of the first steps in using the Facebook Marketing API is to authenticate your account. This is done by generating an access token, which allows your application to make API requests on behalf of your user. Here is an example of how to generate an access token in JavaScript:
FB.getLoginStatus(function(response) {
if (response.status === 'connected') {
var accessToken = response.authResponse.accessToken;
// use the access token to make API requests
} else {
FB.login();
}
});
Creating a Campaign
Once you have authenticated your account, you can begin creating advertising campaigns. Here is an example of how to create a campaign using the Facebook Marketing API in JavaScript:
FB.api('/act_AD_ACCOUNT_ID/adcampaigns', 'POST', {
name: 'My Campaign',
objective: 'LINK_CLICKS',
status: 'PAUSED',
buying_type: 'AUCTION',
promoted_object: {
website_url: 'https://www.example.com'
}
}, function(response) {
console.log(response);
});
Targeting Specific Audiences
The Facebook Marketing API also allows you to target specific audiences for your advertising. Here is an example of how to create a custom audience and use it to target your ads:
FB.api('/act_AD_ACCOUNT_ID/customaudiences', 'POST', {
name: 'My Custom Audience',
subtype: 'WEBSITE',
rule: {
url: {
i_contains: 'example.com'
}
}
}, function(response) {
var audienceId = response.id;
FB.api('/act_AD_ACCOUNT_ID/adsets', 'POST', {
name: 'My Ad Set',
targeting: {
custom_audiences: [{
id: audienceId
}]
}
}, function(response) {
console.log(response);
});
});
Retrieving Insights
Finally, you can use the Facebook Marketing API to retrieve insights about your advertising performance. Here is an example of how to retrieve insights for a specific ad campaign:
FB.api('/AD_CAMPAIGN_ID/insights', {
fields: 'spend, impressions, clicks',
time_range: {
since: '2021-01-01',
until: '2021-01-31'
}
}, function(response) {
console.log(response);
});
Conclusion
In this article, we have covered some basic examples of how to use the Facebook Marketing API in JavaScript. The Facebook Marketing API is a powerful tool that can help developers and marketers create more effective advertising campaigns and integrate their applications with Facebook's ecosystem. If you want to learn more, visit the official documentation website and start exploring the possibilities of the API.