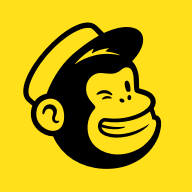
Mailchimp API
MarketingIntroduction to the Mailchimp API
Mailchimp is a popular email marketing tool used by businesses of all sizes to manage their email campaigns. The Mailchimp API is a powerful tool that allows you to integrate Mailchimp capabilities into your own applications, such as syncing your contacts or creating and scheduling campaigns.
This documentation provides a comprehensive list of the Mailchimp API endpoints, with detailed descriptions and examples to help you get started.
API Key Authentication
Before using the Mailchimp API, you will need to obtain an API key. This key will authenticate your requests and allow you to access your Mailchimp account. Your API key should be kept confidential, as it provides access to sensitive information.
To use the Mailchimp API in JavaScript, you can use the fetch()
function and append your API key as a header. Here is an example of how to make a request to the Mailchimp API in JavaScript:
const url = "https://<dc>.api.mailchimp.com/3.0/lists";
const apiKey = "<your-api-key>";
fetch(url, {
headers: {
Authorization: `apikey ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Note that the API key should be appended with the prefix apikey
. The <dc>
in the URL refers to the data center where your Mailchimp account is located. You can find this information in your Mailchimp account settings.
Example API Endpoints
Here are some example API endpoints and example code for using them in JavaScript:
List All Lists
This endpoint returns all the lists in your Mailchimp account.
Endpoint: GET /lists
fetch("https://<dc>.api.mailchimp.com/3.0/lists", {
headers: {
Authorization: `apikey ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Add a New Email to a List
This endpoint adds a new subscriber to a specified list.
Endpoint: POST /lists/{list_id}/members
const listId = "<your-list-id>";
fetch(`https://<dc>.api.mailchimp.com/3.0/lists/${listId}/members`, {
method: "POST",
headers: {
Authorization: `apikey ${apiKey}`,
"Content-Type": "application/json"
},
body: JSON.stringify({
email_address: "john@example.com",
status: "subscribed"
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Create a Campaign
This endpoint creates a new campaign in your Mailchimp account.
Endpoint: POST /campaigns
fetch("https://<dc>.api.mailchimp.com/3.0/campaigns", {
method: "POST",
headers: {
Authorization: `apikey ${apiKey}`,
"Content-Type": "application/json"
},
body: JSON.stringify({
type: "regular",
recipients: {
list_id: "<your-list-id>"
},
settings: {
subject_line: "New Campaign",
from_name: "John",
reply_to: "john@example.com"
}
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
The Mailchimp API is a powerful tool for integrating Mailchimp capabilities into your own applications. This documentation provides comprehensive information on the Mailchimp API endpoints and how to use them in JavaScript. With this knowledge, you can create dynamic and customized email campaigns tailored to your business needs.