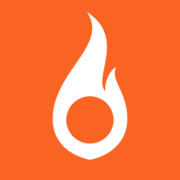
Spark Post API
MarketingExploring the SparkPost Public API Documentation
Are you curious about integrating the SparkPost email delivery service into your application? Getting started with their Public API is easy thanks to the documentation available at https://developers.sparkpost.com/.
In this brief guide, we will explore how to use the SparkPost Public API using JavaScript. But first, let's take a quick look at the basics.
Understanding the SparkPost Public API
The SparkPost Public API allows you to send email, manage recipients, and retrieve message events, among other functionalities. To get started, you will need an API key, which you can obtain by creating a free account on the SparkPost website.
Once you have an API key, you can access the API endpoints by sending HTTP requests to the corresponding URLs. Each endpoint has a specific purpose and accepts different parameters. The SparkPost documentation provides detailed information on each endpoint, including the supported parameters, expected responses, and examples.
Using the SparkPost Public API with JavaScript
To use the SparkPost Public API with JavaScript, we will make use of the built-in fetch
method to send HTTP requests. Let's take a look at a few examples.
Sending an Email
To send an email using the SparkPost Public API, we need to send a POST request to the /api/v1/transmissions
endpoint. Here's an example code snippet that sends a simple email:
// define the API endpoint and your API key
const endpoint = 'https://api.sparkpost.com/api/v1/transmissions';
const apiKey = 'MY_API_KEY';
// define the email message
const message = {
content: {
from: 'sender@example.com',
subject: 'Hello from SparkPost!',
text: 'This is a test email sent using the SparkPost API.'
},
recipients: [
{ address: 'recipient@example.com' }
]
};
// send the email using the fetch method
fetch(endpoint, {
method: 'POST',
headers: {
Authorization: apiKey,
'Content-Type': 'application/json'
},
body: JSON.stringify(message)
})
.then(response => console.log('Email sent!', response))
.catch(error => console.error('Error sending email:', error));
Retrieving Message Events
To retrieve message events using the SparkPost Public API, we need to send a GET request to the /api/v1/events/message
endpoint. Here's an example code snippet that retrieves the events for a specific email message:
// define the API endpoint and your API key
const endpoint = 'https://api.sparkpost.com/api/v1/events/message';
const apiKey = 'MY_API_KEY';
// define the message ID you want to retrieve events for
const messageId = '123456789';
// send the request using the fetch method
fetch(`${endpoint}?message_id=${messageId}`, {
method: 'GET',
headers: {
Authorization: apiKey
}
})
.then(response => response.json())
.then(data => console.log('Message events retrieved!', data))
.catch(error => console.error('Error retrieving events:', error));
Conclusion
With the examples above, you should now have a basic understanding of how to use the SparkPost Public API with JavaScript. Be sure to read through the full documentation to explore the other endpoints and parameters available to you.
We hope this guide has been useful, happy coding!