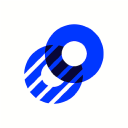
Optimizely
MarketingIntroduction to Optimizely's Public API
If you are a developer looking to improve your website's user experience and conversion rates, you may be interested in using Optimizely's public API. This API allows you to programmatically interact with your Optimizely account and data, making it easier to create and manage experiments, personalization campaigns, and more. In this blog post, we will explore the basics of the Optimizely API and provide some example code in JavaScript.
Getting Started with the Optimizely API
Before you can start using the Optimizely API, you will need to obtain an API token. This can be done through your Optimizely account by following these steps:
- Log in to your Optimizely account.
- Navigate to the "Settings" page.
- Click on the "Access Management" tab.
- Click on the "Create Token" button.
- Give your token a name and select the appropriate permissions.
- Click on the "Create and View" button.
- Copy the token that is displayed.
Once you have your API token, you can start using the Optimizely API. The base URL for the API is https://api.optimizely.com/v2/
. All API requests must be sent over HTTPS.
Example API Requests in JavaScript
Here are some example API requests that you can make using JavaScript:
Get a List of Projects
To get a list of all your Optimizely projects, you can make a GET request to /projects
. Here is an example in JavaScript:
fetch('https://api.optimizely.com/v2/projects', {
headers: {
'Authorization': 'Bearer <Your API Token>'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Create a New Experiment
To create a new experiment in Optimizely, you can make a POST request to /experiments
. Here is an example in JavaScript:
fetch('https://api.optimizely.com/v2/experiments', {
method: 'POST',
headers: {
'Authorization': 'Bearer <Your API Token>',
'Content-Type': 'application/json'
},
body: JSON.stringify({
'project_id': '<Your Project ID>',
'status': 'running',
'description': 'This is my new experiment',
'type': 'ab',
'variations': [
{
'actions': [
{
'type': 'javascript',
'value': 'console.log("Hello, world!")'
}
],
'name': 'Variation 1'
},
{
'actions': [
{
'type': 'javascript',
'value': 'console.log("Goodbye, world!")'
}
],
'name': 'Variation 2'
}
]
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Update an Experiment
To update an existing experiment in Optimizely, you can make a PATCH request to /experiments/{experiment_id}
. Here is an example in JavaScript:
fetch('https://api.optimizely.com/v2/experiments/123456789', {
method: 'PATCH',
headers: {
'Authorization': 'Bearer <Your API Token>',
'Content-Type': 'application/json'
},
body: JSON.stringify({
'description': 'This is my updated experiment',
'status': 'paused'
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
The Optimizely API provides a powerful way to programmatically interact with your Optimizely account and data. With just a few lines of code in JavaScript, you can create and manage experiments, personalization campaigns, and more. If you are interested in learning more about the Optimizely API, be sure to check out the official documentation and get started today!