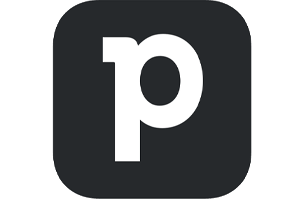
Pipedrive API
MarketingWith Pipedrive CRM designed for salespeople, by salespeople, you’re able to do more to grow your business. Easily build integrations with our API and reach over 90k companies in the Pipedrive Marketplace. Pipedrive is a sales CRM with an intuitive RESTful API. You can use our API to create public or private apps using OAuth and integrations via API token. Public apps and integrations work directly with Pipedrive and are available in the Pipedrive's Marketplace.
📚 Documentation & Examples
Everything you need to integrate with Pipedrive API
🚀 Quick Start Examples
// Pipedrive API API Example
const response = await fetch('https://developers.pipedrive.com/docs/api/v1/#/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Introduction to Pipedrive API
Pipedrive offers an API that you can use to interact with your Pipedrive CRM data from any programming language. This API documentation provides detailed information about how to use the API, including example code in JavaScript.
Getting Started
Before you can start using the Pipedrive API, you will need to create a Pipedrive account and obtain your API key. Your API key is used to authenticate your API requests. Once you have your API key, you are ready to start making API calls.
Examples
The following are some example API calls that you can make using the Pipedrive API.
List Deals
To list all deals in Pipedrive, use the following code:
fetch('https://api.pipedrive.com/v1/deals?api_token=YOUR_API_TOKEN')
.then(response => response.json())
.then(data => console.log(data))
Create a Deal
To create a new deal in Pipedrive, use the following code:
fetch('https://api.pipedrive.com/v1/deals?api_token=YOUR_API_TOKEN', {
method: 'POST',
body: JSON.stringify({
title: 'New Deal',
value: '100.00',
currency: 'USD',
person_id: '123'
}),
headers: {
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data))
Update a Deal
To update an existing deal in Pipedrive, use the following code:
fetch('https://api.pipedrive.com/v1/deals/123?api_token=YOUR_API_TOKEN', {
method: 'PUT',
body: JSON.stringify({
title: 'Updated Deal',
value: '200.00',
currency: 'USD'
}),
headers: {
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data))
Conclusion
The Pipedrive API provides a powerful way to interact with your CRM data programmatically. With this documentation and example code, you should be able to start integrating the Pipedrive API into your applications.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes