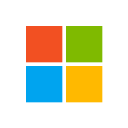
Microsoft Advertising Platform - Bing Ads API
MarketingIntroduction to Microsoft Advertising API
Microsoft Advertising API is a web-based API that enables developers to work with Microsoft Advertising data programmatically. With this API, developers can automate bulk operations, such as creating campaigns, ad groups, keywords, and ads, without using the UI. The API service is provided by Microsoft Advertising, formerly known as Bing Ads.
In this article, we will discuss how to use the Microsoft Advertising API, starting with creating a developer account, obtaining API credentials, and building a simple JavaScript application.
Prerequisites
Before using the Microsoft Advertising API, you will need to create a developer account and obtain API credentials. You can create a developer account by following these steps:
- Go to Microsoft Advertising developer center
- Click on "Sign in" and enter your Microsoft account credentials.
- Click on "Create a new account" to create a new Microsoft Advertising account if you don't have one.
- Click on "My Account" and then click on "API Access".
- Follow the instructions to create an API application and obtain API credentials.
Using the Microsoft Advertising API
Once you have your API credentials, you can start using the Microsoft Advertising API in JavaScript. Here is an example of how to authenticate with the API and retrieve account information:
const clientId = 'your-client-id';
const clientSecret = 'your-client-secret';
const refreshToken = 'your-refresh-token';
const accountId = 'your-advertising-account-id';
const fetchToken = async () => {
const response = await fetch('https://login.microsoftonline.com/common/oauth2/v2.0/token', {
method: 'post',
body: `client_id=${clientId}&client_secret=${clientSecret}&refresh_token=${refreshToken}&grant_type=refresh_token`
});
if (response.ok) {
const json = await response.json();
const token = json.access_token;
return token;
}
throw new Error('Failed to fetch access token');
};
const getAccount = async () => {
const token = await fetchToken();
const response = await fetch(`https://ads.microsoft.com/api/advertiser/v1/accounts/${accountId}`, {
headers: {
'Authorization': `Bearer ${token}`
}
});
if (response.ok) {
const json = await response.json();
return json;
}
throw new Error('Failed to fetch account information');
};
(async () => {
const account = await getAccount();
console.log(account);
})();
In this example, we define four variables: clientId
, clientSecret
, refreshToken
, and accountId
. We then define a function fetchToken
that retrieves an access token using the clientId
, clientSecret
, and refreshToken
. We use this access token to authenticate the API request to retrieve the account information.
To retrieve the account information, we define a function getAccount
that makes a GET request to the /accounts/{accountId}
endpoint of the Microsoft Advertising API with the token
in the Authorization
header.
Finally, we call the getAccount
function using an async IIFE (Immediately Invoked Function Expression) and log the returned account information to the console.
Conclusion
In this article, we introduced the Microsoft Advertising API and discussed how to use it to retrieve account information programmatically. We used JavaScript code to authenticate with the API and make a GET request to retrieve the account information. There are many more endpoints and operations that can be performed using the Microsoft Advertising API, and we encourage you to explore the API documentation to learn more.