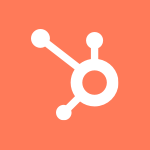
Hubspot
MarketingHubSpot API Overview
The HubSpot API is a powerful tool that allows developers to integrate their applications with HubSpot's platform. With HubSpot's API, developers can get access to contact and company data, create and manage deals, and much more.
In this blog post, we'll provide an overview of the HubSpot API and cover some example code using JavaScript.
Getting Started
Before you can start using the HubSpot API, you'll need to create a developer account and obtain an API key. To create a developer account, navigate to the HubSpot Developer Dashboard. Once you've created an account, you can generate an API key by following the instructions here.
API Endpoints
The HubSpot API is organized into different endpoints that correspond to different types of data. Some of the most commonly used endpoints include:
- Contacts API: Used to access contact information such as name, email, and phone number.
- Companies API: Used to access company information such as name, location, and industry.
- Deals API: Used to create and manage deals within HubSpot.
- Engagements API: Used to manage activities such as calls, emails, and meetings.
Example Code
Here are some examples of how you can use the HubSpot API with JavaScript:
Retrieving Contact Properties
const axios = require('axios');
const API_KEY = 'INSERT_API_KEY_HERE';
axios.get(`https://api.hubapi.com/contacts/v1/properties?hapikey=${API_KEY}`)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Creating a New Company
const axios = require('axios');
const API_KEY = 'INSERT_API_KEY_HERE';
const company = {
name: 'New Company',
industry: 'Technology',
city: 'San Francisco',
state: 'CA',
zip: '94107',
domain: 'newcompany.com'
};
axios.post(`https://api.hubapi.com/companies/v2/companies?hapikey=${API_KEY}`, company)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Updating a Contact's Email Address
const axios = require('axios');
const API_KEY = 'INSERT_API_KEY_HERE';
const contactId = '123';
const newEmail = 'newemail@example.com';
axios.patch(`https://api.hubapi.com/contacts/v1/contact/vid/${contactId}/profile?hapikey=${API_KEY}`, {
"properties": [
{
"property": "email",
"value": newEmail
}
]
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Conclusion
The HubSpot API is a powerful tool that can open up many possibilities for developers. In this blog post, we covered some of the basics of the HubSpot API and provided some example code using JavaScript.
If you're interested in learning more about the HubSpot API, be sure to check out the official documentation.