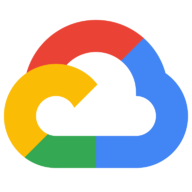
Google Maps API
GeocodingExploring the Google Maps Platform API Documentation
Are you looking to integrate Google Maps functionality into your web application? Look no further than the Google Maps Platform API! With a variety of APIs available for location-based services, you can add maps, directions, and more to your web app with ease.
To get started, head over to the Google Maps Platform website and create a free account. Once you've done that, you'll have access to a variety of APIs, including Maps JavaScript API, Geocoding API, Directions API, and more.
Maps JavaScript API
Let's start with the Maps JavaScript API, which allows you to display maps on your web page and customize them with markers, infowindows, and other features.
Getting started
To use the Maps JavaScript API, you'll need to include the API script in your HTML file:
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY"></script>
Replace YOUR_API_KEY
with the API key you generated on the Google Cloud Console. Once the script is included, you can create a map by adding the following code to your JavaScript file:
function initMap() {
const map = new google.maps.Map(document.getElementById("map"), {
zoom: 8,
center: { lat: 37.7749, lng: -122.4194 },
});
}
This creates a map centered on San Francisco with a zoom level of 8. To display the map on your web page, add a <div>
element with the id
attribute set to "map":
<div id="map"></div>
Adding markers and infowindows
Now that you have a map, let's add some markers and infowindows to it. You can create markers and infowindows by adding the following code to your initMap()
function:
const marker = new google.maps.Marker({
position: { lat: 37.7749, lng: -122.4194 },
map: map,
});
const infowindow = new google.maps.InfoWindow({
content: "San Francisco",
});
marker.addListener("click", () => {
infowindow.open(map, marker);
});
This creates a marker at the center of the map and an infowindow with the content "San Francisco". When the marker is clicked, the infowindow will be displayed.
Customizing the map style
You can customize the style of the map by adding a styles
option to the map constructor:
const map = new google.maps.Map(document.getElementById("map"), {
zoom: 8,
center: { lat: 37.7749, lng: -122.4194 },
styles: [
{
featureType: "water",
elementType: "geometry",
stylers: [{ color: "#e9e9e9" }, { lightness: 17 }],
},
// Add more styles here
],
});
This example changes the color of the water to a light grey and adjusts the lightness to 17 for a softer look.
Geocoding API
The Geocoding API allows you to convert addresses into geographic coordinates (latitude and longitude) and vice versa.
Getting started
To use the Geocoding API, you'll need to include the API script in your HTML file:
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY"></script>
Replace YOUR_API_KEY
with the API key you generated on the Google Cloud Console. Once the script is included, you can use the Geocoder
class to geocode an address:
const geocoder = new google.maps.Geocoder();
geocoder.geocode({ address: "1600 Amphitheatre Parkway, Mountain View, CA" }, (results, status) => {
if (status === "OK") {
console.log(results[0].geometry.location); // {lat: 37.4219999, lng: -122.0840575}
} else {
console.error("Geocode was not successful for the following reason: " + status);
}
});
This example geocodes the address "1600 Amphitheatre Parkway, Mountain View, CA" and logs the latitude and longitude of the result to the console.
Reverse geocoding
You can also use the Geocoding API to perform reverse geocoding, which converts geographic coordinates into an address.
const geocoder = new google.maps.Geocoder();
geocoder.geocode({ location: { lat: 37.7749, lng: -122.4194 } }, (results, status) => {
if (status === "OK") {
console.log(results[0].formatted_address); // "San Francisco, CA, USA"
} else {
console.error("Reverse geocode was not successful for the following reason: " + status);
}
});
This example performs reverse geocoding on the coordinates for San Francisco and logs the formatted address to the console.
Directions API
The Directions API allows you to retrieve directions between two or more locations.
Getting started
To use the Directions API, you'll need to include the API script in your HTML file:
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY"></script>
Replace YOUR_API_KEY
with the API key you generated on the Google Cloud Console. Once the script is included, you can use the DirectionsService
and DirectionsRenderer
classes to display directions on a map:
const directionsService = new google.maps.DirectionsService();
const directionsRenderer = new google.maps.DirectionsRenderer();
directionsRenderer.setMap(map);
directionsService.route(
{
origin: "Chicago, IL",
destination: "Los Angeles, CA",
travelMode: "DRIVING",
},
(response, status) => {
if (status === "OK") {
directionsRenderer.setDirections(response);
} else {
console.error("Directions request failed due to " + status);
}
}
);
This example retrieves driving directions from Chicago to Los Angeles and displays them on the map.
Summary
The Google Maps Platform API offers a wealth of functionality for web developers looking to incorporate location-based services into their web applications. From displaying maps and markers to performing geocoding and retrieving directions, the API has everything you need to create a dynamic and engaging user experience.
Happy mapping!