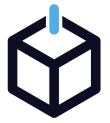
Holiday Oracle
Data AccessDiscover the World of Holidays with Holiday Oracle API
Are you looking for a reliable API to fetch data about holidays worldwide? Look no further than the Holiday Oracle API. The API provides access to an extensive database of holidays across the globe, including regional and national holidays.
Getting Started with the Holiday Oracle API
To get started with the Holiday Oracle API, you need to sign up for an API key on the website. You can sign up for a free account that offers up to 100 requests per month, or a paid account that gives you access to more requests and features.
After obtaining your API key, you can start making requests to the API endpoints. The API provides endpoints for fetching holidays by country, region, and specific dates.
Fetching Holidays by Country
You can use the following JavaScript code to fetch holidays by country using the Holiday Oracle API:
const apiKey = 'your_api_key';
const countryCode = 'us'; // change to your desired country code
fetch(`https://api.holidayoracle.io/v1/holidays/country/${countryCode}?key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
The above code fetches holidays for the United States using the API key provided. You can change the countryCode
variable to fetch holidays for any other country supported by the API.
Fetching Holidays by Region
To fetch holidays by region, you can use the following JavaScript code:
const apiKey = 'your_api_key';
const countryCode = 'us'; // change to your desired country code
const regionCode = 'ca'; // change to your desired region code
fetch(`https://api.holidayoracle.io/v1/holidays/country/${countryCode}/region/${regionCode}?key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
In the above code, the countryCode
variable indicates the country, and the regionCode
variable indicates the region code within the country. You can change these variables to fetch holidays for any other country and region combination supported by the API.
Fetching Holidays by Date Range
To fetch holidays within a specific date range, you can use the following JavaScript code:
const apiKey = 'your_api_key';
const countryCode = 'us'; // change to your desired country code
const startDate = '2022-01-01'; // change to your desired start date
const endDate = '2022-01-07'; // change to your desired end date
fetch(`https://api.holidayoracle.io/v1/holidays/country/${countryCode}/date/${startDate}/${endDate}?key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
In the above code, the startDate
and endDate
variables indicate the date range for which you want to fetch holidays. The date format should be in YYYY-MM-DD
format. You can change the countryCode
, startDate
, and endDate
variables to fetch holidays for any other country and date range supported by the API.
Conclusion
The Holiday Oracle API provides a simple and reliable way to fetch data about holidays worldwide. With easy-to-use endpoints, you can quickly and efficiently fetch the information you need for your application or service.