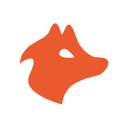
Hunter
Data AccessExploring the Hunter.io API Documentation
Hunter.io is a powerful tool that allows you to search for and verify email addresses on a large scale. The Hunter.io API is a great way to integrate this functionality into your own applications and tools, and this documentation will provide you with everything you need to get started.
Getting Started
The first thing you'll need to do is create an account on Hunter.io and generate an API key. Once you have your API key, you can start using the API.
All examples in this blog post will be written using JavaScript, but the Hunter.io API is also compatible with other programming languages.
Searching for Email Addresses
One of the key features of Hunter.io is its ability to search for email addresses associated with a particular domain. Here's an example of how to use the API to do this:
const fetch = require('node-fetch');
const domain = 'example.com';
const apiKey = 'your_api_key';
fetch(`https://api.hunter.io/v2/domain-search?domain=${domain}&api_key=${apiKey}`)
.then(res => res.json())
.then(data => {
console.log(data);
})
.catch(err => {
console.error(err);
});
In this example, we're using the node-fetch
package to make an HTTP request to the Hunter.io API. We pass in our API key and the domain we're interested in, and the API will return a JSON object containing information about all the email addresses associated with that domain.
Verifying Email Addresses
Another useful feature of Hunter.io is its ability to verify email addresses to ensure that they're valid and active. Here's an example of how to use the API to do this:
const fetch = require('node-fetch');
const email = 'john.doe@example.com';
const apiKey = 'your_api_key';
fetch(`https://api.hunter.io/v2/email-verifier?email=${email}&api_key=${apiKey}`)
.then(res => res.json())
.then(data => {
console.log(data);
})
.catch(err => {
console.error(err);
});
This example is very similar to the previous one, but instead of passing in a domain, we're passing in an email address. The API will return a JSON object containing information about that email address, including whether or not it's a valid and active email address.
Conclusion
The Hunter.io API is a powerful tool that can be used to search for and verify email addresses on a large scale. With the examples provided in this blog post, you should be well on your way to integrating the API into your own applications and tools. If you have any questions or need further assistance, be sure to refer to the comprehensive documentation provided by Hunter.io.