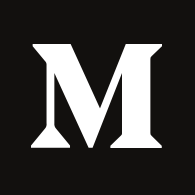
Medium
Data AccessExploring the Medium API with NoCodeAPI
NoCodeAPI provides a suite of APIs to help developers build web applications without coding. One of the most useful APIs in the NoCodeAPI suite is the Medium API. With this API, developers can easily retrieve data from the Medium platform.
In this blog post, we will explore the different functionalities of the Medium API and learn how to use its features in JavaScript using NoCodeAPI.
Getting started with NoCodeAPI
The first step to using NoCodeAPI is to create an account and generate your unique API key. Once you have your API key, you can start using the NoCodeAPI suite to connect to different APIs, including the Medium API.
To connect to the Medium API, you can use the following code in JavaScript:
const key = "YOUR_API_KEY_HERE";
const mediumApiUrl = `https://api.nocodeapi.com/medium-api?apiKey=${key}`;
In the code above, we first define our API key as a constant variable. We then define the URL for the Medium API endpoint, including our API key as a query parameter.
Retrieving blog posts
One of the key functionalities of the Medium API is to retrieve blog posts from the platform. To do this, we can use the following code:
fetch(mediumApiUrl)
.then((response) => response.json())
.then((data) => {
console.log(data); // This will display the blog posts in JSON format in the console
});
The code above sends a GET request to the Medium API endpoint, retrieves the data returned from the endpoint as a JSON object, and logs it to the console.
Filtering blog posts
If you want to filter the blog posts returned from the Medium API endpoint, you can add query parameters to the URL. For example, to retrieve only blog posts with the tag "JavaScript", you can use the following URL:
const mediumApiUrl = `https://api.nocodeapi.com/medium-api?apiKey=${key}&tag=javascript`;
You can also filter by author, publication, and time range. Here is an example code to retrieve blog posts from a certain publication:
const mediumApiUrl = `https://api.nocodeapi.com/medium-api?apiKey=${key}&publication=techcrunch`;
Retrieving blog post details
In addition to retrieving a list of blog posts, you can also retrieve details about a specific blog post. To do this, you can add the post ID as a query parameter to the Medium API endpoint URL. Here is an example code:
const postId = "POST_ID_HERE";
const mediumApiUrl = `https://api.nocodeapi.com/medium-api?apiKey=${key}&postId=${postId}`;
fetch(mediumApiUrl)
.then((response) => response.json())
.then((data) => {
console.log(data); // This will display the blog post details in JSON format in the console
});
Conclusion
The Medium API is a powerful tool for developers looking to retrieve data from the Medium platform. With NoCodeAPI, it is easy to connect to the Medium API and retrieve blog posts and related information. Use the code examples in this post to get started with the Medium API and start building your own web applications!