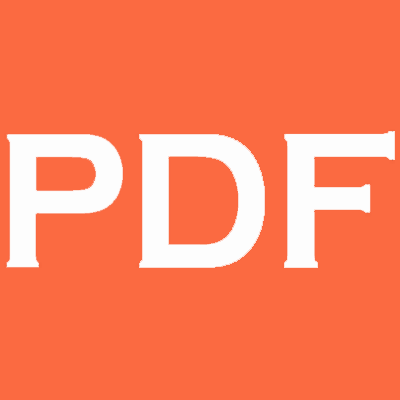
PDF Merge
Data AccessPDF Merge API - A Reliable Way to Merge PDFs
The PDF Merge API is a powerful tool that allows users to merge multiple PDF files into a single document. With this API, developers can easily integrate PDF merging functionality into their applications. In this article, we will explore this API and provide examples of it's functionality using JavaScript code.
Getting Started
To get started with the PDF Merge API, you will need to sign up for an account to obtain your API key. Once you have your API key, you can start using the API.
Merging PDFs
Merging PDFs with this API is a breeze. Simply make a POST request to the API endpoint and provide the PDFs you wish to merge as parameters. Here's an example code in JavaScript:
const apiKey = "[Your API Key]";
const sourceFiles = [
"https://website.com/file1.pdf",
"https://website.com/file2.pdf",
"https://website.com/file3.pdf"
];
const requestOptions = {
method: 'POST',
headers: { 'content-type': 'application/json', 'x-api-key': apiKey },
body: JSON.stringify({ url: sourceFiles.join(",") })
};
fetch('https://api.pdf.co/v1/pdf/merge', requestOptions)
.then(response => response.json())
.then(result => console.log(result))
.catch(error => console.log('error', error));
This code creates a POST request to the API endpoint, providing an array of URLs to the PDF files to merge. Once the API processes the request, the merged PDF file will be returned as a result.
Customizing PDF Merge
The PDF Merge API also provides several customization options to enhance the merged PDF file. You can specify options such as password protection, page orientation, and page size. Here's an example of how to customize the PDF merge using JavaScript code:
const apiKey = "[Your API Key]";
const sourceFiles = [
"https://website.com/file1.pdf",
"https://website.com/file2.pdf",
"https://website.com/file3.pdf"
];
const requestOptions = {
method: 'POST',
headers: { 'content-type': 'application/json', 'x-api-key': apiKey },
body: JSON.stringify({
url: sourceFiles.join(","),
password: "test",
orientation: "Landscape",
pageSize: "A4"
})
};
fetch('https://api.pdf.co/v1/pdf/merge', requestOptions)
.then(response => response.json())
.then(result => console.log(result))
.catch(error => console.log('error', error));
In this example, we're specifying that we want the merged PDF file to be password protected, landscape-oriented, and A4-sized.
Conclusion
The PDF Merge API is a reliable and powerful tool that developers can use to merge PDF files in their applications. With the ability to customize the output, users can create professional and highly-functional PDF documents. By using the JavaScript code examples provided above, you can quickly and easily integrate this API into your applications.