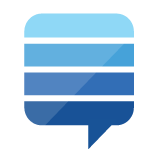
StackExchange
DevelopmentExploring Stack Exchange with Public API
As developers, we all love a good API, don't we? The Stack Exchange Public API is one such glorious API that lets us interact with the data on Stack Exchange sites like Stack Overflow, Super User, and others.
To get started with the API, you first need to register your app on the Stack Apps website to obtain an API key. Once you have the API key, you are ready to interact with the API.
Retrieving Questions
To retrieve questions from Stack Overflow, you can use the /questions
endpoint. Here's how you can do that in JavaScript:
const apiKey = 'YOUR_API_KEY_HERE';
const stackOverflowURL = `https://api.stackexchange.com/2.2/questions?&pagesize=10&order=desc&sort=votes&site=stackoverflow&key=${apiKey}`;
fetch(stackOverflowURL)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are retrieving the top 10 questions from Stack Overflow, sorted by votes in descending order.
Searching for Questions
To search for questions, you can use the /search
endpoint. Here's how to perform a search for "JavaScript":
const apiKey = 'YOUR_API_KEY_HERE';
const stackOverflowURL = `https://api.stackexchange.com/2.2/search?&pagesize=10&order=desc&sort=votes&intitle=javascript&site=stackoverflow&key=${apiKey}`;
fetch(stackOverflowURL)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are searching for the top 10 questions on Stack Overflow that contain the word "JavaScript" in their title, sorted by votes in descending order.
Retrieving Answers for a Question
To retrieve answers for a question, you can use the /questions/{ids}/answers
endpoint. Here's how to retrieve answers for a question with ID 123456
:
const apiKey = 'YOUR_API_KEY_HERE';
const questionId = '123456';
const stackOverflowURL = `https://api.stackexchange.com/2.2/questions/${questionId}/answers?&order=desc&sort=votes&site=stackoverflow&key=${apiKey}`;
fetch(stackOverflowURL)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are retrieving the answers for a question with ID 123456
on Stack Overflow, sorted by votes in descending order.
Conclusion
The Stack Exchange Public API is a powerful tool for developers who want to interact with Stack Exchange sites. In this article, we covered some examples in JavaScript for retrieving questions, searching for questions, and retrieving answers. Give it a try, and explore what the API has to offer!