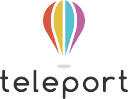
Teleport
Data AccessExploring Teleport API with JavaScript
Teleport is an open data platform that lets you explore cities around the world. The API endpoints are available for developers to use in their applications. In this article, we will take a look at how to use the Teleport API with JavaScript and explore its various features.
Getting Started
To use the Teleport API, you need to sign up for an API key. Once you have your API key, you can start making requests to the API endpoints. There are multiple endpoints available to explore different information about cities such as urban area details, location details, score, and ranks.
In the following example, we will use the https://api.teleport.org/api/cities/
endpoint to get a list of all available cities in the Teleport platform.
const apiKey = 'your_api_key';
const apiUrl = `https://api.teleport.org/api/cities/?search=`;
fetch(apiUrl, {
headers: {
Authorization: `Bearer ${apiKey}`,
Accept: 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data._embedded["city:search-results"]))
.catch(error => console.error(error));
The above code uses fetch()
method to make a GET request to the Teleport API. We have added an Authorization
header with our API key to authorize the request. After parsing the response using .json()
method, we are logging the list of the cities to the console.
Exploring Urban Area Details
Teleport API provides detailed information on urban areas. In the following example, we will use the https://api.teleport.org/api/urban_areas/slug:
endpoint to get the details of an urban area based on its slug.
const urbanAreaSlug = 'san-francisco-bay-area';
const apiUrl = `https://api.teleport.org/api/urban_areas/slug:${urbanAreaSlug}`;
fetch(apiUrl, {
headers: {
Authorization: `Bearer ${apiKey}`,
Accept: 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
The above code will fetch data based on the slug san-francisco-bay-area
, which will return the details of the San Francisco Bay Area urban area. You can also change the urbanAreaSlug
variable according to your preference.
Conclusion
In conclusion, Teleport API provides a great way to explore detailed information related to different cities and urban areas. With the help of JavaScript, developers can easily fetch this information and use it in their applications. We have seen how to use Teleport API with JavaScript and explored two of its endpoints. You can always check out the official documentation for more information. Happy coding!