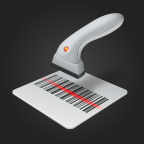
UPC database
Data AccessUsing the UPC Database API in JavaScript
If you're looking for a public API that provides information on products, the UPC Database API is a great option. With this API, you can retrieve information such as product names, descriptions, and images based on a product's UPC.
Here's how you can get started using the UPC Database API in JavaScript.
Getting an API Key
To use the UPC Database API, you'll need to sign up for an account and get an API Key. Once you have an API Key, you can start making requests.
Making a Request
To retrieve information on a product, you'll need to make a request to the UPC Database API's lookup
endpoint. Here's an example request:
const apiKey = 'your_api_key';
const upc = '012345678901';
fetch(`https://api.upcitemdb.com/prod/trial/lookup?upc=${upc}`, {
headers: {
'Content-Type': 'application/json',
'x-api-key': apiKey
}
})
.then(response => response.json())
.then(data => console.log(data));
In the example above, we're using the fetch
function to make a GET request to the UPC Database API's lookup
endpoint. The x-api-key
header is where we pass in our API Key. We're also passing in a UPC code in the request URL.
Handling the Response
After making a request, the UPC Database API will return a JSON response containing information on the requested product. Here's an example response:
{
"code": "012345678901",
"total": 1,
"offset": 0,
"items": [
{
"ean": "0123456789012",
"title": "Example Product",
"description": "Lorem ipsum dolor sit amet, consectetur adipiscing elit.",
"brand": "Example Brand",
"images": [
"https://example.com/product-image.jpg"
],
"offers": [
{
"merchant": "Example Merchant",
"currency": "USD",
"price": "19.99",
"link": "https://example.com/product"
}
]
}
]
}
To access the information in the response, you can simply use object notation. For example, to get the product title from the response, you can use the following code:
const title = data.items[0].title;
Conclusion
Using the UPC Database API in JavaScript is simple, thanks to its easy-to-use interface. With its robust product information, this API can be incredibly useful in building product-oriented applications.