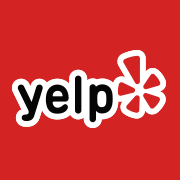
π Documentation & Examples
Everything you need to integrate with Yelp
π Quick Start Examples
// Yelp API Example
const response = await fetch('https://www.yelp.com/developers/documentation/v3', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Yelp API Documentation
Are you looking for a comprehensive API documentation for the Yelp website? Look no further than https://www.yelp.com/developers/documentation/v3. The website provides a wide range of endpoints that enable developers to access Yelp's vast database of businesses and reviews.
API Examples in JavaScript
To help you get started with Yelp API, we have compiled some code examples in JavaScript. These examples demonstrate the process of calling Yelp APIs using different endpoints.
API Key
Before you can use Yelp API, you need to obtain an API key. The API key is a unique identifier that allows you to access Yelp's database and make API calls. Visit https://www.yelp.com/login?return_url=%2Fdevelopers%2Fv3%2Fmanage_app to obtain your API key.
Search Endpoint
The search endpoint provides businesses based on search filters like location, tags, price range, and more. The following example shows how to call the search endpoint using JavaScript.
const apiKey = 'YOUR_API_KEY_HERE';
const url = 'https://api.yelp.com/v3/businesses/search';
const formData = {
term: 'cream puffs',
location: 'San Francisco',
price: '2,3',
sort_by: 'rating'
};
const headers = {
Authorization: `Bearer ${apiKey}`
};
fetch(url, {
method: 'POST',
headers,
body: JSON.stringify(formData)
})
.then(response => response.json())
.then(data => console.log(data));
Phone Endpoint
The phone endpoint returns businesses based on the provided phone number. The following code example shows how to call the phone endpoint using JavaScript.
const apiKey = 'YOUR_API_KEY_HERE';
const url = 'https://api.yelp.com/v3/businesses/search/phone';
const formData = {
phone: '+14157492060'
};
const headers = {
Authorization: `Bearer ${apiKey}`
};
fetch(`${url}/${formData.phone}`, {
headers
})
.then(response => response.json())
.then(data => console.log(data.businesses));
Business Endpoint
The business endpoint provides detailed information about a specific business based on the provided business ID. The following code example shows how to call the business endpoint using JavaScript.
const apiKey = 'YOUR_API_KEY_HERE';
const url = 'https://api.yelp.com/v3/businesses';
const businessId = 'meR6bzAQDxK81Lj-54pLgQ';
const headers = {
Authorization: `Bearer ${apiKey}`
};
fetch(`${url}/${businessId}`, {
headers
})
.then(response => response.json())
.then(data => console.log(data));
Conclusion
In conclusion, the Yelp API provides developers with a vast database of businesses and reviews to create innovative applications. The documentation provides clear examples in JavaScript to help developers implement the API endpoints effectively. Get started today and leverage Yelp's API to create engaging applications!
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes