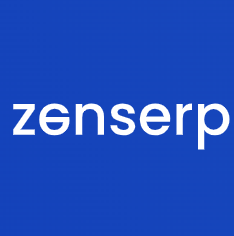
Zenscrape
Data AccessScraping Made Easy with ZenScrape API
Looking for an easy and efficient way to scrape website data? Look no further than ZenScrape! With our powerful API, you can quickly and easily extract data from any website in just a few lines of JavaScript code.
Getting Started with ZenScrape
First, you'll need to sign up for a ZenScrape API key. You can do this by visiting our website and following the prompts to create an account. Once you've signed up, you'll be given an API key that you can use to make requests to our API.
Next, you'll need to choose the endpoint you want to use. We offer a wide range of endpoints designed to provide you with all the data you need.
Sample API Code
To get started with ZenScrape API in JavaScript, simply use the following sample code:
const apiKey = 'YOUR_API_KEY';
const endpoint = 'ENDPOINT_NAME';
const url = 'https://www.example.com';
const headers = new Headers();
headers.append('apikey', apiKey);
fetch(`https://api.zenscrape.com/v1/${endpoint}?url=${url}`, {
method: 'GET',
headers: headers,
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
Make sure to replace 'YOUR_API_KEY' with your own API key, 'ENDPOINT_NAME' with the endpoint you want to use (e.g. 'html', 'text', 'image', etc.), and 'https://www.example.com' with the URL of the website you want to scrape.
Example Endpoints
Here are a few examples of the endpoints we offer:
HTML Endpoint
const apiKey = 'YOUR_API_KEY';
const endpoint = 'html';
const url = 'https://www.example.com';
const headers = new Headers();
headers.append('apikey', apiKey);
fetch(`https://api.zenscrape.com/v1/${endpoint}?url=${url}`, {
method: 'GET',
headers: headers,
})
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.log(error));
Text Endpoint
const apiKey = 'YOUR_API_KEY';
const endpoint = 'text';
const url = 'https://www.example.com';
const headers = new Headers();
headers.append('apikey', apiKey);
fetch(`https://api.zenscrape.com/v1/${endpoint}?url=${url}`, {
method: 'GET',
headers: headers,
})
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.log(error));
Image Endpoint
const apiKey = 'YOUR_API_KEY';
const endpoint = 'image';
const url = 'https://www.example.com';
const headers = new Headers();
headers.append('apikey', apiKey);
fetch(`https://api.zenscrape.com/v1/${endpoint}?url=${url}`, {
method: 'GET',
headers: headers,
})
.then(response => response.blob())
.then(blob => console.log(URL.createObjectURL(blob)))
.catch(error => console.log(error));
Conclusion
With ZenScrape API, web scraping has never been easier. Whether you're looking to extract data from HTML pages, text files, or images, our powerful endpoints are designed to help you get the information you need quickly and easily. With a little bit of JavaScript code, you can begin using our API today and start reaping the benefits of effortless web scraping!