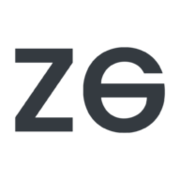
Zenserp
Data AccessFast Search Result Scraping with our SERP API Our SERP API enables you to scrape search engine result pages in realtime. Get started with just a few clicks by signing up for our free plan.
π Documentation & Examples
Everything you need to integrate with Zenserp
π Quick Start Examples
// Zenserp API Example
const response = await fetch('https://zenserp.com', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Zenserp API Docs
Zenserp is an API service that provides search engine result pages (SERPs) to businesses and developers. The API provides JSON formatted SERPs for Google, Bing, and Yahoo search engines.
Authentication
To access the Zenserp API, you need to sign up for an account and obtain an API key. Once you have your API key, you need to include it in the headers of your API requests as follows:
const api_key = 'YOUR_API_KEY'
const options = {
headers: {
'apikey': api_key
}
}
Usage
Basic Usage
Here is a basic example of how to use the Zenserp API to retrieve Google search results for a keyword in JavaScript:
const axios = require('axios')
const api_key = 'YOUR_API_KEY'
const search_query = 'puppy'
const options = {
headers: {
'apikey': api_key
},
params: {
q: search_query,
search_engine: 'google.com'
}
}
axios.get('https://app.zenserp.com/api/v2/search', options)
.then(response => {
console.log(response.data)
})
.catch(error => {
console.log(error)
})
Advanced Usage
You can also use the Zenserp API to retrieve more detailed SERP information by including additional parameters in your API requests. Here is an example of how to retrieve Google News search results:
const axios = require('axios')
const api_key = 'YOUR_API_KEY'
const search_query = 'apple'
const options = {
headers: {
'apikey': api_key
},
params: {
q: search_query,
search_engine: 'google.com',
tbm: 'nws'
}
}
axios.get('https://app.zenserp.com/api/v2/search', options)
.then(response => {
console.log(response.data)
})
.catch(error => {
console.log(error)
})
Conclusion
In conclusion, the Zenserp API is a powerful tool for accessing search engine result pages for Google, Bing, and Yahoo search engines. It is easy to use and can be integrated into any project with JavaScript support. To learn more about the Zenserp API, visit their website.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes