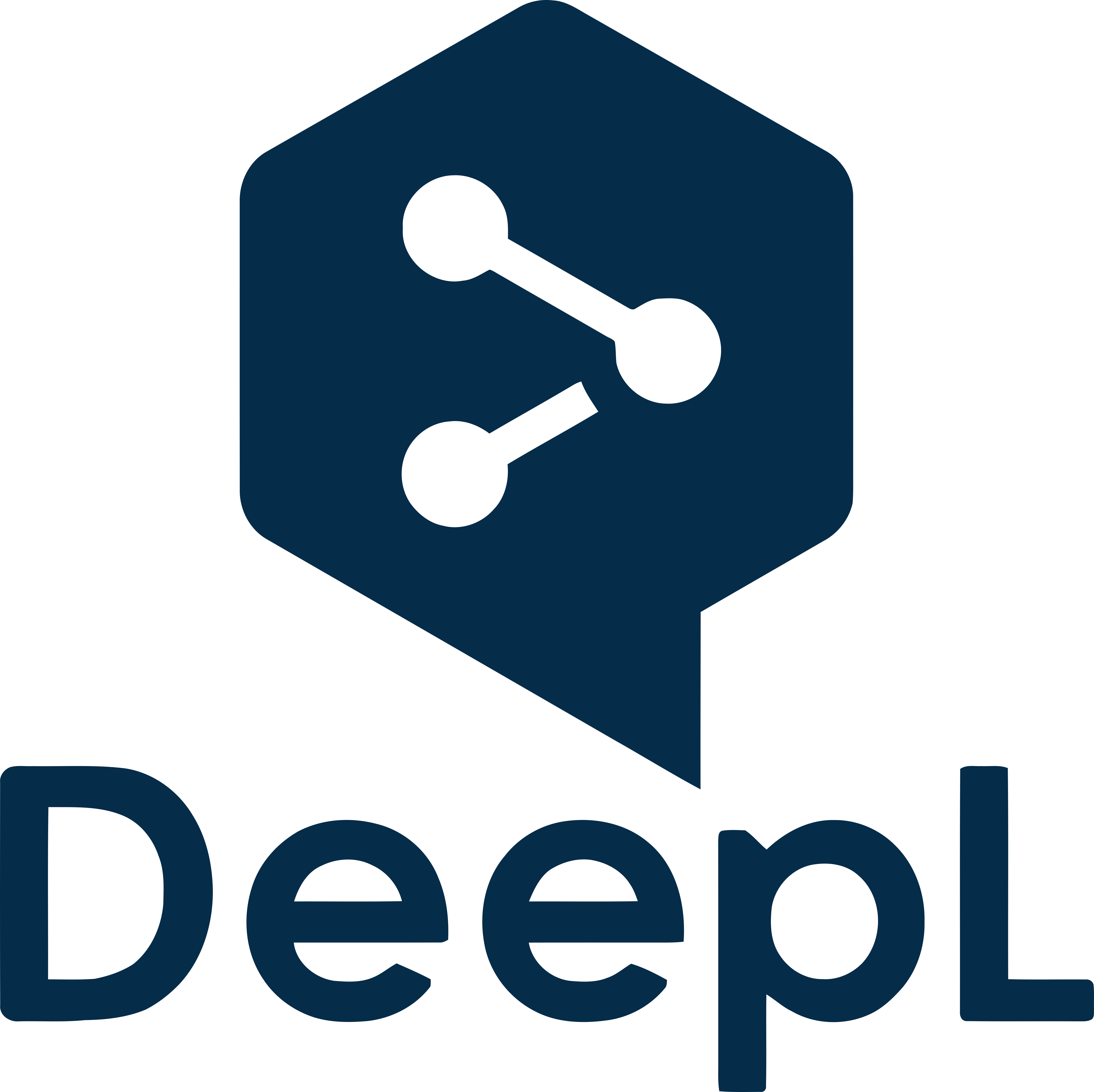
DeepL API
MediaA Guide to DeepL API
DeepL API is a language translation tool that can be integrated into various applications. It provides a RESTful API that can be accessed using several programming languages. In this guide, we will focus on JavaScript and explore some basic usage examples.
Before You Start
Before you begin using the DeepL API, you need to obtain an API key. You can get your API key by creating an account on their website and subscribing to their API plan. You can choose from different pricing plans based on your usage requirements.
Installation
To use the API in your JavaScript code, you need to install the axios package. Axios is a promise-based HTTP client for the browser and node.js. You can install it using npm:
npm install axios
Getting Started
Once you have your API key and axios installed, you can start using the API. The API endpoint is https://api.deepl.com/v2/translate
. You need to provide your API key as the auth_key
parameter. You also need to provide the text you want to translate as the text
parameter, and the target language as the target_lang
parameter.
Here's an example:
const axios = require('axios');
const API_KEY = 'your_api_key_here';
const URL = 'https://api.deepl.com/v2/translate';
async function translateText(text, targetLang) {
const { data } = await axios.post(URL, {
auth_key: API_KEY,
text,
target_lang: targetLang,
});
return data.translations[0].text;
}
// Example usage:
translateText('Hello, how are you?', 'DE').then((result) => {
console.log(result);
});
In this example, we're defining a function called translateText
that takes two parameters: text
and targetLang
. It uses axios to post a request to the DeepL API endpoint, passing in the API key, text, and target language as parameters. The function returns the translated text from the API response.
You can use this function to translate any text to your desired language. The target language is specified as a two-letter code.
Advanced Usage
The DeepL API also supports several optional parameters that can be used to customize the translation process. For example, you can specify the source language using the source_lang
parameter. You can also specify the formality using the formality
parameter.
Here's an example code snippet that includes these optional parameters:
async function translateTextAdvanced(text, targetLang, sourceLang = 'EN', formality = 'default') {
const { data } = await axios.post(URL, {
auth_key: API_KEY,
text,
target_lang: targetLang,
source_lang: sourceLang,
formality: formality,
});
return data.translations[0].text;
}
// Example usage:
translateTextAdvanced('Hello, how are you?', 'DE', 'EN', 'more').then((result) => {
console.log(result);
});
In this example, we've defined a new function that includes the optional parameters sourceLang
and formality
. The sourceLang
parameter specifies the source language, which defaults to English. The formality
parameter specifies the level of formality, which defaults to default
.
You can experiment with different values for these parameters to get the best translation results.
Conclusion
In this guide, we've explored the basics of using the DeepL API in JavaScript. We've seen how to obtain an API key, install axios, and use the API in your code. We've also seen how to customize the translation process using optional parameters.
The DeepL API can be a powerful tool for language translation in many use cases. By following the examples in this guide, you should be able to get started quickly and easily. Happy translating!