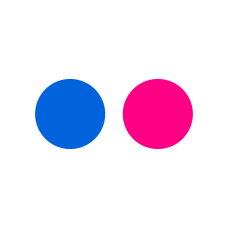
Flickr
MediaIntroduction
APIs or Application Programming Interfaces are a way for computer systems and applications to interact with each other. Flickr, a popular photo-sharing site, has an API that allows developers to access photos and information from the site. In this blog post, we will look at some examples of using the Flickr API in JavaScript.
Setting Up
Before we begin, we need to set up our development environment. We will need to get an API key from Flickr in order to access the API. To get an API key, we need to create a Flickr account and then go to the Flickr API website.
Once we have our API key, we can start using the API.
Example 1: Search Photos
The first example we will look at is searching for photos on Flickr. We will use the flickr.photos.search
method to search for photos that match a specific keyword.
Here is an example code snippet:
const apiKey = "YOUR_API_KEY";
const url = "https://api.flickr.com/services/rest/";
const searchBtn = document.getElementById("searchBtn");
searchBtn.addEventListener("click", searchPhotos);
function searchPhotos() {
const query = document.getElementById("searchInput").value;
const requestUrl = url + "?method=flickr.photos.search&api_key=" + apiKey + "&text=" + query + "&format=json&nojsoncallback=1";
fetch(requestUrl)
.then(response => response.json())
.then(data => {
const photos = data.photos.photo;
const photoContainer = document.getElementById("photoContainer");
photoContainer.innerHTML = "";
photos.forEach(photo => {
const imgSrc = `https://live.staticflickr.com/${photo.server}/${photo.id}_${photo.secret}_q.jpg`;
const imgElement = document.createElement("img");
imgElement.setAttribute("src", imgSrc);
photoContainer.appendChild(imgElement);
});
});
}
In this code snippet, we get the search keyword from an input field on our page. We then construct the request URL and make a fetch request to the Flickr API. Once we get the response back, we extract the photos from the response and display them on our page.
Example 2: Get Photo Information
The second example we will look at is getting information about a specific photo. We will use the flickr.photos.getInfo
method to get information such as the title, description, and tags of a photo.
Here is an example code snippet:
const apiKey = "YOUR_API_KEY";
const url = "https://api.flickr.com/services/rest/";
const photoId = "PHOTO_ID";
const requestUrl = url + "?method=flickr.photos.getInfo&api_key=" + apiKey + "&photo_id=" + photoId + "&format=json&nojsoncallback=1";
fetch(requestUrl)
.then(response => response.json())
.then(data => {
const photo = data.photo;
console.log(photo.title._content);
console.log(photo.description._content);
console.log(photo.tags.tag);
});
In this code snippet, we specify the photo ID of the photo we want to get information about. We then construct the request URL and make a fetch request to the Flickr API. Once we get the response back, we extract the information we need and log it to the console.
Conclusion
In this blog post, we looked at two examples of using the Flickr API in JavaScript. We saw how to search for photos and how to get information about a specific photo. The Flickr API has many other methods that allow us to do other things such as uploading photos and getting information about users. You can find more information about the Flickr API on the Flickr API website.