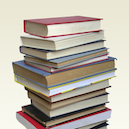
Good Reads
MediaExploring the Goodreads API
Goodreads is a popular platform for book lovers. It allows users to create virtual bookshelves, discover new titles, and interact with other readers. Goodreads also has an open API that developers can use to create their own applications. This blog post will explore the Goodreads API and provide some examples of how to use it in JavaScript.
Requirements
To get started, you will need an API key from Goodreads. You can sign up for a key here. Once you have your key, you can start making requests to the API.
Getting Started
Let's start with a simple example. We will use the search.books
method to search for books that contain the word "javascript" in the title. We will call the API using the GET
method and pass our API key and search parameters as query parameters.
const apiKey = 'YOUR_API_KEY'; // Replace with your actual API key
const searchQuery = 'javascript';
fetch(`https://www.goodreads.com/search/index.xml?key=${apiKey}&q=${searchQuery}`)
.then(response => response.text())
.then(data => {
var parser = new DOMParser();
var xmlData = parser.parseFromString(data, "text/xml");
console.log(xmlData);
})
.catch(error => console.log(error));
In this code, we are using the fetch
method to make a GET request to the Goodreads API. We pass our API key and search query as query parameters in the URL. We then use the then()
method to parse the response as XML data using DOMParser
.
Working with the API Responses
Goodreads API returns the data in XML format. You can use the DOMParser
to parse the XML, as we did in the previous example. Once you have the parsed data, you can extract the information you need using querySelector
or querySelectorAll
.
Here is an example of how to extract book titles and authors from the search results:
const books = xmlData.querySelectorAll('search > results > work');
books.forEach(book => {
const title = book.querySelector('best_book > title').textContent;
const author = book.querySelector('best_book > author > name').textContent;
console.log(`${title} by ${author}`);
});
In this code, we are using querySelector
to select the title
and author
elements from each work
node in the XML data. We then use the textContent
property to get the actual text content of these elements.
Conclusion
Goodreads API is a great resource for building applications that involve books. In this post, we explored how to use the API in JavaScript, including making API calls and parsing the XML response. If you want to learn more about the API, check out the official documentation.