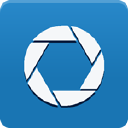
screenshotlayer API
MediaIntroduction to the Screenshotlayer API
The Screenshotlayer API allows you to capture high-quality screenshots of any website in real-time. Simply provide the URL of the website and receive a screenshot in PNG format. The API is easy-to-use and accessible through a simple REST API.
Getting Started
To use the Screenshotlayer API, you need to have an API access key. You can sign up for a free account on the Screenshotlayer website to get your API key.
API Endpoint
The base URL for the API is http://api.screenshotlayer.com/api/capture
. All requests to the API should include your API access key as a query parameter.
For example:
http://api.screenshotlayer.com/api/capture?access_key=YOUR_ACCESS_KEY&url=https://www.example.com
API Parameters
The Screenshotlayer API supports a range of parameters that allow you to customize the screenshot output. Here are some of the most commonly used parameters:
url: The URL of the website to be captured.
viewport: The size of the viewport in pixels. The format is width x height
.
fullpage: Capture the entire webpage, not just the visible part.
width: The width of the output screenshot in pixels.
delay: Wait for a specific number of seconds before capturing the screenshot.
ttl: Set the time-to-live for the screenshot in seconds.
force: Override the cache and force a new screenshot to be captured.
Example API Call in JavaScript
Here's an example of how to make an API call to Screenshotlayer using JavaScript:
const apiKey = "YOUR_ACCESS_KEY";
const url = "https://www.example.com";
const viewport = "1280x720";
const apiUrl = `http://api.screenshotlayer.com/api/capture?access_key=${apiKey}&url=${url}&viewport=${viewport}`;
fetch(apiUrl)
.then(response => response.blob())
.then(data => {
const img = document.createElement('img');
img.src = URL.createObjectURL(data);
document.body.appendChild(img);
})
.catch(error => console.error(error));
This code creates an API URL from the access key, URL, and viewport size and makes a fetch
request to the API. The response is then converted to a blob and displayed on the page as an image.
Conclusion
The Screenshotlayer API is a powerful tool for capturing high-quality screenshots of any webpage. With a range of customizable parameters, you can easily tailor your screenshots to suit your specific needs.