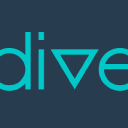
TasteDive
MediaDiscover New Things with TasteDive API
TasteDive is a recommendation engine that helps users find new things to explore - from movies, TV shows, music, books, authors, games, and podcasts.
The TasteDive API provides developers with a way to query their extensive database and retrieve recommendations for various categories.
Getting Started
Before we start, you need to get an API key from the TasteDive website. Once you have the key, you can make requests to their API.
Here is the endpoint URL you would use to make requests:
https://tastedive.com/api/similar
Making Queries
To retrieve recommendations, you need to provide the name of the item you want to search for, as well as other parameters such as type, limit, and info.
Here is an example code snippet in JavaScript that retrieves recommendations for the movie "The Lion King":
const fetch = require('node-fetch');
const API_KEY = 'YOUR_API_KEY';
const URL = `https://tastedive.com/api/similar?q=The%20Lion%20King&type=movie&k=${API_KEY}&info=1&limit=5`;
fetch(URL)
.then(response => response.json())
.then(data => console.log(data.Similar.Results))
.catch(error => console.error(error));
Some important things to note:
node-fetch
is a module that makes it easy to fetch resources over HTTP or HTTPSAPI_KEY
is the key you obtained from the TasteDive websiteq
is the parameter that specifies the search item. In this case, it's "The Lion King"type
is the parameter that specifies the type of item. In this case, it's "movie"info
is the parameter that specifies the amount of detail to returnlimit
is the parameter that specifies the maximum number of results to return
Response Data
The response data from TasteDive API is a JSON object that contains an array of similar recommendations.
Here is an example response for "The Lion King":
{
"Similar": {
"Info": [
{
"Name": "The Lion King",
"Type": "movie",
"wTeaser": "The Lion King is a 1994 American animated musical film produced by Walt Disney Feature Animation and released by Walt Disney Pictures. It is the 32nd Disney animated feature film, and the fifth animated film produced during a period known as the Disney Renaissance. The Lion King tells the story of Simba, a young lion who is to succeed his father, Mufasa, as king of the Pride Lands; however, after Simba's uncle Scar kills Mufasa, Simba is manipulated into thinking he was responsible and flees into exile. Upon returning years later, he must regain his place as king and deal with Scar.",
"wUrl": "https://en.wikipedia.org/wiki/The_Lion_King",
"yUrl": "https://www.youtube.com/watch?v=4sj1MT05lAA",
"yID": "4sj1MT05lAA",
"yEmbed": "<iframe width=\"480\" height=\"360\" src=\"https://www.youtube.com/embed/4sj1MT05lAA?feature=oembed\" frameborder=\"0\" allowfullscreen></iframe>"
}
],
"Results": [
{
"Name": "The Lion King 2: Simba's Pride",
"Type": "movie"
},
{
"Name": "Aladdin",
"Type": "movie"
},
{
"Name": "Beauty and the Beast",
"Type": "movie"
}
]
}
}
The Similar.Results
field contains an array of similar items, along with their types.
Conclusion
The TasteDive API is a powerful tool for discovering new things in various categories. With just a few lines of code, you can retrieve recommendations for any search item you want.
Try building your own application using the TasteDive API and see what interesting recommendations you can discover!