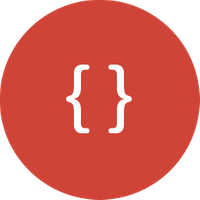
The Open Movie Database
MediaA Beginner's Guide to Using OMDB API with JavaScript
OMDB API is a free RESTful web service that provides comprehensive movie and TV series data. The API is designed to allow developers to access and retrieve useful information such as movie titles, descriptions, ratings, and more. With the following guide, we will explore how to make API requests using JavaScript.
API Authentication
The first step to using OMDB API is to request an API key. A key serves as a unique identifier that allows the API to track your usage and serve the data back to you. To get a valid API key, go to http://www.omdbapi.com/, register, and receive your own API key.
API Requests
After you have obtained a valid API key, you can start making requests to the OMDB API.
Basic Request
The most straightforward request to the API looks like this:
http://www.omdbapi.com/?apikey=<YOUR_API_KEY>&t=<MOVIE_TITLE>
where <YOUR_API_KEY>
represents your OMDB API key, and <MOVIE_TITLE>
represents the title of the movie you want to retrieve.
For example, to retrieve data for a movie "The Shawshank Redemption", replace <YOUR_API_KEY>
with your API key and <MOVIE_TITLE>
with the real title of the movie.
http://www.omdbapi.com/?apikey=<YOUR_API_KEY>&t=The+Shawshank+Redemption
Now, let's see how we can use JavaScript to make a request to the API.
Making Requests with JavaScript
We will use fetch
to make requests to the API. Run the following code in your browser console to get the movie data to console:
const api_key = <YOUR_API_KEY>;
const movie_title = 'The Shawshank Redemption';
fetch(`https://www.omdbapi.com/?apikey=${api_key}&t=${movie_title}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This script will make a GET request to OMDB API and return the movie data in JSON format. Once you obtain the movie data, you can use this data to display useful information on your web page.
Handling API Responses
Once your request is successful, the API will send back a JSON object with all the movie data you requested. You can use this data to populate your HTML with useful information such as movie title, poster image, rating, and more.
Suppose you want to display the movie title and plot. Add the following code snippet to your script:
const api_key = <YOUR_API_KEY>;
const movie_title = 'The Shawshank Redemption';
fetch(`https://www.omdbapi.com/?apikey=${api_key}&t=${movie_title}`)
.then(response => response.json())
.then(data => {
const title = data.Title;
const plot = data.Plot;
console.log(title, plot);
})
.catch(error => console.error(error));
Conclusion
In this tutorial, we have shown you how to use OMDB API with JavaScript to retrieve movie data. We have explored the basics of API authentication, making requests, handling responses with JSON, and how to display useful movie information on your web page.
Use this guide as a starting point to begin exploring the many possibilities provided by OMDB API. Start building your movie website today!