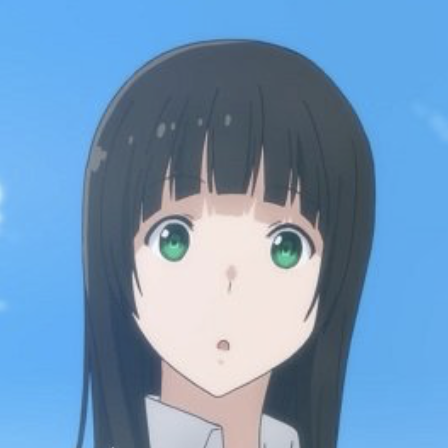
Trace.moe API
MediaUsing the Trace.moe Public API in JavaScript
If you're looking for a powerful public API for anime and manga content, look no further than Trace.moe. This API makes it easy to analyze and extract information from video or image files, so you can quickly identify key details about specific anime and manga scenes.
Let's take a closer look at how to use the Trace.moe API in JavaScript.
Getting Started with Trace.moe
Before you can start using the Trace.moe API, you'll need to sign up for an API key. You can do this by visiting the Trace.moe website and creating an account.
Once you have your API key, you can start making requests using your preferred programming language. In this case, we'll be using JavaScript.
Making Requests with JavaScript
To use the Trace.moe API in JavaScript, you'll first need to install the axios
library. This library makes it easy to send HTTP requests and handle responses.
Here's an example of how to use the Trace.moe API in JavaScript:
const axios = require('axios');
const endpoint = 'https://trace.moe/api/search';
const apiKey = 'your api key here';
// Example search parameters
const params = {
image: '', // Base64-encoded image data
url: '', // URL of image file
anime: '', // Title of anime series
anilist_id: '', // Anilist ID of anime series
episode: '', // Episode number
filename: '', // Filename of video file
video: '', // URL of video file
limit: '', // Maximum number of results to return
similarity: '', // Minimum similarity score
start_time: '', // Start time of clip (in seconds)
end_time: '' // End time of clip (in seconds)
};
axios.get(endpoint, {
params,
headers: {
'x-access-token': apiKey
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
In this example, we're making a GET request to the Trace.moe API endpoint and passing in our search parameters as query parameters. We're also including our API key in the x-access-token
header.
Once we receive a response, we're logging the JSON data to the console.
Conclusion
The Trace.moe public API is a powerful tool for working with anime and manga content. With a few lines of JavaScript, you can easily search and analyze video and image files to extract valuable information.
By following the example code above, you can quickly get started with using the Trace.moe API in your own projects.