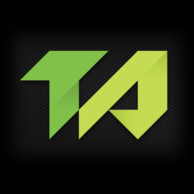
TrailerAddict
MediaExploring the TrailerAddict API
TrailerAddict provides a public API for developers to access its movie trailers database. The API allows you to search for movie trailers, get information about movies, and access other related data. In this blog post, we will explore the TrailerAddict API and provide some example code in JavaScript.
Getting Started
Before we begin, you will need to sign up for an API key from TrailerAddict in order to use their API. After you have obtained a key, you can start making requests to the API.
API Endpoints
The TrailerAddict API has several endpoints that allow you to get different types of data about movies. Let's take a look at some of them:
Search for a Movie Trailer
If you want to search for a movie trailer, you can use the following endpoint:
https://api.traileraddict.com/v4/search/movie
To make a request to this endpoint, you will need to pass in a q
parameter with the movie name or keyword you want to search for. Here's an example of how you can search for the trailer of the movie "Inception" using JavaScript:
const apiKey = 'your_api_key';
const movieName = 'inception';
fetch(`https://api.traileraddict.com/v4/search/movie?q=${movieName}&count=1&width=460&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
Get Movie Information
If you want to get information about a specific movie, you can use the following endpoint:
https://api.traileraddict.com/v4/movie/info
To make a request to this endpoint, you will need to pass in a imdb
parameter with the IMDb ID of the movie you want to get information about. Here's an example of how you can get information about the movie "Inception" using JavaScript:
const apiKey = 'your_api_key';
const imdbId = 'tt1375666';
fetch(`https://api.traileraddict.com/v4/movie/info?imdb=${imdbId}&width=460&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
Get Movie Trailer Embed Code
If you want to get the embed code for a movie trailer, you can use the following endpoint:
https://api.traileraddict.com/v4/trailer/embed
To make a request to this endpoint, you will need to pass in a movie
parameter with the IMDb ID of the movie you want to get the trailer for. Here's an example of how you can get the trailer embed code for the movie "Inception" using JavaScript:
const apiKey = 'your_api_key';
const imdbId = 'tt1375666';
fetch(`https://api.traileraddict.com/v4/trailer/embed?imdb=${imdbId}&width=460&autoplay=y&apikey=${apiKey}`)
.then(response => response.text())
.then(data => console.log(data));
Conclusion
In this blog post, we explored the TrailerAddict API and provided some example code in JavaScript. We looked at how to search for a movie trailer, get information about a movie and get the trailer embed code. The TrailerAddict API provides a lot of useful data for developers who are looking to build movie-related applications. Happy coding!