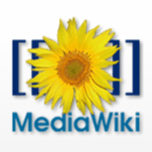
Wikipedia
MediaIntroduction to MediaWiki API
MediaWiki is a free and open-source software wiki package written in PHP. The MediaWiki API is a collection of web services that allow developers to access and manipulate data from Wikimedia projects like Wikipedia, Wiktionary, Wikidata, etc. The API provides an interface for retrieving data from these projects in multiple formats like JSON, XML, and HTML. In this blog, we will explore the MediaWiki API and how to use it to access Wikimedia project data using JavaScript.
Getting Started
To use the MediaWiki API, you need to authenticate yourself by obtaining an API token. This token is used to identify your API requests and ensure that only authorized clients can access the API. To obtain an API token, you need to have an account on one of the Wikimedia projects.
Once you have an API token, you can start using the MediaWiki API by sending HTTP requests to the API endpoint. The API endpoint for the MediaWiki API is https://en.wikipedia.org/w/api.php
, where en.wikipedia.org
can be replaced with the name of any Wikimedia project.
Example Code
Here are some example API calls in JavaScript using the fetch()
method:
Get page content by title
fetch('https://en.wikipedia.org/w/api.php?action=query&prop=extracts&titles=Albert%20Einstein&format=json')
.then(response => response.json())
.then(data => console.log(data.query.pages))
.catch(error => console.error(error));
Search for page titles
fetch('https://en.wikipedia.org/w/api.php?action=query&list=search&srsearch=moon&format=json')
.then(response => response.json())
.then(data => console.log(data.query.search))
.catch(error => console.error(error));
Get random page title
fetch('https://en.wikipedia.org/w/api.php?action=query&generator=random&grnnamespace=0&prop=info&inprop=url&grnlimit=1&format=json')
.then(response => response.json())
.then(data => console.log(data.query.pages))
.catch(error => console.error(error));
The fetch()
method sends a GET request to the specified API endpoint. The then()
method processes the response and logs the resulting data to the console.
Conclusion
The MediaWiki API is a powerful tool for accessing data from Wikimedia projects. With the help of JavaScript and the fetch()
method, you can easily interact with the API and retrieve the data you need. This blog has covered only a few of the many features and capabilities of the MediaWiki API, so be sure to check out the API documentation for more information.